Table of Contents
18. Table tile¶
A table tile inherit from renderizable and implement all methods as usual. Also the animation has a shader table by default which can be accessed by getShader method.
A tile is created using the tile map editor
18.1. tile map definitions¶
There are some definitions that are important to understand.
18.1.1. Brick ID¶
Brick ID is a unique identifier for each brick.
In the editor the brick ID is just a number of itself:
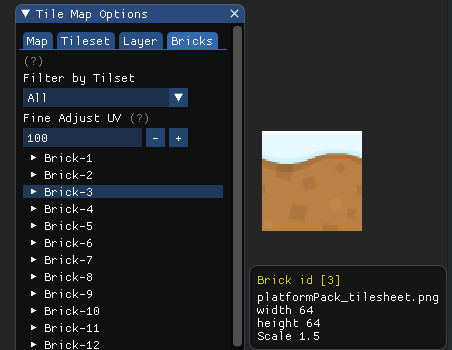
Figure 18.1 Brick ID on editor¶
18.1.2. Tile ID in the map¶
This is how tile are organized in the map.
It is called ‘tile’ when the brick it is in the map.
The total of tile is count width tile x count height tile.
The first tile is the ID 1, and the last one depends on map size.
If we have for example 20x10 tiles we will have 200 tiles in total. 200th is the last one.
Zero means no tile .
See the figure to understand better:
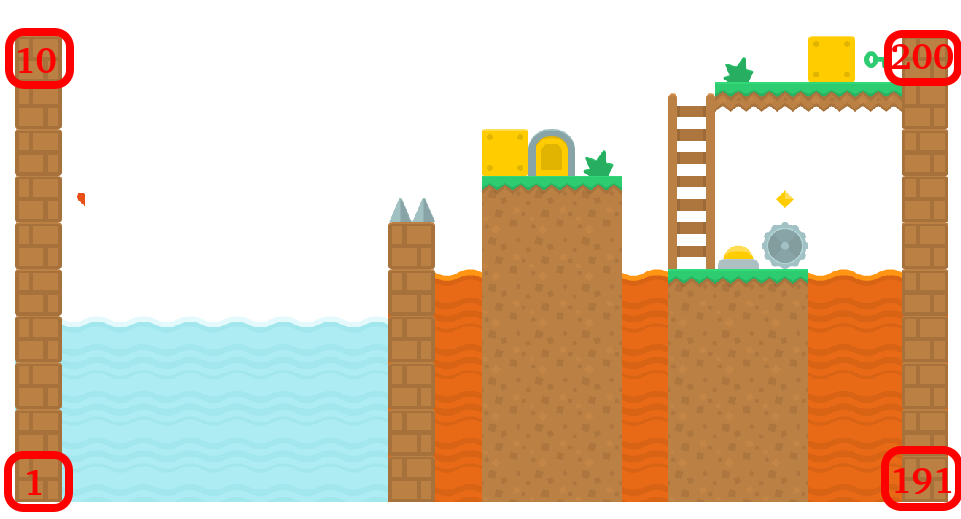
Figure 18.2 20x10 tiles = 200 tiles.¶
18.2. tile methods¶
18.2.1. tile new¶
- new(string * world, number * x, number * y, number * z)¶
Create a new instance of a tile passing the world desired (detail) and position.
- Parameters
string – world can be
2ds
,2dw
or3d
.number – x position (optional).
number – y position (optional).
number – z position (optional).
- Returns
tile table.
Example:
tTile = tile:new('2dw')
18.2.2. tile load¶
- load(string file_name)¶
Load a tile from file (expected
.tile
extension).- Parameters
string – file name from file.
Example:
tTile = tile:new('2dW')
if tTile:load('my_map.tile') then
print('Successfully loaded tile map:','my_map.tile')
else
print('Failed to loaded tile map:','my_map.tile')
end
Note
18.2.3. tile setLayerVisible¶
- setLayerVisible(number layer, boolean value)¶
Turn a layer visible or not indicating the number of it (one based).
- Parameters
number – layer 1 to total of layer.
boolean – visible
true
orfalse
.
Example:
tTile = tile:new('2dW')
if tTile:load('my_map.tile') then
tTile:setLayerVisible(1,false)
else
print('Failed to loaded tile map:','my_map.tile')
end
Note
18.2.4. tile getTotalLayer¶
- getTotalLayer¶
Retrieve the total of layer from tile map.
- Returns
number
- total of layer.
Example:
tTile = tile:new('2dW')
if tTile:load('my_map.tile') then
print('Total layer:',tTile:getTotalLayer())
else
print('Failed to loaded tile map:','my_map.tile')
end
18.2.5. tile getTypeMap¶
- getTypeMap¶
Retrieve the type of the tile map (
isometric
,orthogonal
,hexagonal
)- Returns
string
- type of tile map.
Example:
tTile = tile:new('2dW')
if tTile:load('my_map.tile') then
print('Type Tile Map:',tTile:getTypeMap())
else
print('Failed to loaded tile map:','my_map.tile')
end
18.2.6. tile getTileID¶
- getTileID(number x, number y, number layer)¶
Retrieve the tile ID in the map given
x
,y
in2d screen
coordinate and the layer number.- Parameters
number – x coordinate in
2d screen
.number – y coordinate in
2d screen
.number – layer 1 to total of layer.
- Returns
number
- ID of tile on tile map (1 to total of tile on map).
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
function onTouchMove(key,x,y)
local layer = 1
local ID = tTile:getTileID(x,y,layer)
print('Tile ID:', ID)
end
18.2.7. tile disableTile¶
- disableTile(number x, number y, number * layer)¶
Disable rendering of tile, given the position
x
,y
of the tile on the map in2d screen
coordinate, and the layer number.- Parameters
number – x coordinate in
2d screen
.number – y coordinate in
2d screen
.number – layer 1 to total of layer. (Optional)
- Returns
number
- position of tile on tile map. (Zero means not found atx
,y
coordinate).
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
function onTouchDown(key,x,y)
local ID = tTile:disableTile(x,y)
if ID ~= 0 then
print ('Tile disabled ID ',ID)
end
end
Note
18.2.8. tile enableTile¶
- enableTile(number x, number y, number * layer)¶
Enable rendering of tile, given the position
x
,y
of the tile on the map in2d screen
coordinate, and the layer number.- Parameters
number – x coordinate in
2d screen
.number – y coordinate in
2d screen
.number – layer 1 to total of layer. (Optional)
- Returns
number
- position of tile on tile map. (Zero means not found atx
,y
coordinate).
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
function onTouchDown(key,x,y)
local ID = tTile:enableTile(x,y)
if ID ~= 0 then
print ('Tile Enabled ID ',ID)
end
end
Note
18.2.9. tile getPositionsFromBrickID¶
- getPositionsFromBrickID(number idBrick, number layer)¶
Return a
table
ofx
,y
positions, andlayer
of one or more tiles found on the map, according to the brick ID and the number of layer (can be omitted).- Parameters
number – brick id.
number – layer (one based).
- Returns
table
-{ x, y, layer}, { x, y, layer}, ...}
.
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local brickID = 32 -- according to bricks
local tPos = tTile:getPositionsFromBrickID(brickID)
for i=1, #tPos do
print(i,tPos[i].x,tPos[i].y,tPos[i],tPos[i].layer)
end
18.2.10. tile getPositionFromTileID¶
- getPositionFromTileID(number tileID)¶
Return the
x
,y
tile position given the tile ID on map.- Parameters
number – tile id.
- Returns
number
- x,number
- y
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local tileID = 32
local x,y = tTile:getPositionFromTileID(tileID)
print('Center of tile 32:',x,y)
18.2.11. tile getNearPosition¶
- getNearPosition(number x, number y, number layer)¶
Return the near
x
,y
tile position given x,y in2d screen
and layer.- Parameters
number – x position in
2d screen
.number – y position in
2d screen
.number – layer (one based) (if not supplied will get the first one).
- Returns
number
- x,number
- y
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local x,y = 10, 10
local x,y = tTile:getNearPosition(x,y)
18.2.12. tile setTileID¶
- setTileID(number x, number y, number brickID, number layer)¶
Set a tile to render a brick given the brick ID at
x
,y
position in2d screen
, andlayer
.- Parameters
number – x position in
2d screen
.number – y position in
2d screen
.number – brick ID.
number – layer (one based).
- Returns
boolean
- result of operation.
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
function onTouchDown(key,x,y)
local brickID = 1
local layer = 1
tTile:setTileID(x,y,brickID,layer)
end
18.2.13. tile getTileID¶
- getTileID(number x, number y, number brickID, number layer)¶
Retrieve the tile ID and brick ID (if there is one set) given
x
,y
position in2d screen
, andlayer
.- Parameters
number – x position in
2d screen
.number – y position in
2d screen
.number – layer (one based).
- Returns
number
- tile ID,number
- brick ID.
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
function onTouchDown(key,x,y)
local layer = 1
local tileID, brickID = tTile:getTileID(x,y,layer)
print('tileID', tileID,'brickID', brickID)
end
18.2.14. tile getTileSize¶
- getTileSize¶
Retrieve the default tile size considering the scale.
- Returns
number
- width,number
- height.
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local width,height = tTile:getTileSize()
print('default size of tile:',width,height)
18.2.15. tile getMapSize¶
- getMapSize¶
Retrieve the map size considering the scale.
- Returns
number
- width,number
- height.
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local width,height = tTile:getMapSize()
print('Map size:',width,height)
18.2.16. tile getProperties¶
- getProperties(table * filter)¶
Retrieve a array of a table with properties according to the filter.
- Parameters
table – filter
{name,owner,type,ID}
(might be omitted).- Returns
table
-{{name,value,owner,type}, ...}
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local tProperties = tTile:getProperties({owner = 'layer', type = 'string'})
for i=1, #tProperties do
local tProperty = tProperties[i]
print(tProperty.name,tProperty.value,tProperty.owner,tProperty.type)
end
Note
The filter is optional and when present, one or more fields might be used.
18.2.17. tile getObjects¶
- getObjects(table * filter)¶
Retrieve a array of a table with objects according to the filter.
- Parameters
table – filter
{name,type,ID}
(might be omitted).- Returns
table
-{{name,type, ...}, ...}
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local tRectangles = tTile:getObjects({type = 'rectangle'})
for i=1, #tRectangles do
local tObject = tRectangles[i]
print(tObject.type,tObject.name,tObject.x,tObject.y,tObject.width,tObject.height)
end
local tCircles = tTile:getObjects({type = 'circle'})
for i=1, #tCircles do
local tObject = tCircles[i]
print(tObject.type,tObject.name,tObject.x,tObject.y,tObject.ray)
end
local tTriangles = tTile:getObjects({type = 'triangle'})
for i=1, #tTriangles do
local tObject = tTriangles[i]
print(tObject.type,tObject.name,tObject.p1.x,tObject.p1.y, tObject.p2.x,tObject.p2.y, tObject.p3.x,tObject.p3.y)
end
local tPoints = tTile:getObjects({type = 'point'})
for i=1, #tPoints do
local tObject = tPoints[i]
print(tObject.type,tObject.name,tObject.x,tObject.y)
end
local tPoints = tTile:getObjects({type = 'line'})
for i=1, #tPoints do
local tObject = tPoints[i]
print(' ' .. tObject.type,tObject.name)
for j=1, #tObject.points do
local point = tObject.points[j]
print(point.x,point.y)
end
end
Note
Expected object types:
Type
Fields
rectangle
x
y
width
height
circle
x
y
ray
triangle
p1 = {x,y}
p2 = {x,y}
p1 = {x,y}
point
x
y
line
points = {[1] = {x,y}, [2] = {x,y}, ...}
18.2.18. tile getPhysicsBrick¶
- getPhysicsBrick(number brickID)¶
Retrieve a array of a table with physics from brick as defined in the editor.
- Parameters
number – brickID
- Returns
table
-{{name,type, ...}, ...}
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local brickID = 47
local tPhysics = tTile:getPhysicsBrick(brickID)
for i=1, #tPhysics do
local tObject = tPhysics[i]
print(tObject.type,tObject.name,tObject.x,tObject.y)
end
Note
A physic table might be the same table as object table for rectangle
, circle
and triangle
.
18.2.19. tile createTileObject¶
- createTileObject(number tileID, number layer)¶
Create a independent Tile Object from an existent tile ID given the layer.
The Tile Object will no long be controlled by tile map, however, if the tile map is destroyed, it is also destroyed.
This Tile Object is inherited from renderizable.
- Parameters
number – tileID
number – layer
- Returns
table
-renderizable
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local tileID = 144
local layer = 2
tSaw = tTile:createTileObject(tileID,layer)
function loop(delta)
tSaw.az = tSaw.az + math.rad(50)
end
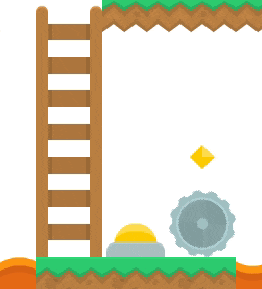
Figure 18.3 Using tile object as normal renderizable after building it.¶
18.2.20. tile setLayerZ¶
- setLayerZ(number layer, number z)¶
Set
z
position oflayer
.- Parameters
number – layer (one based).
number – z position.
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local layer, z = 1, 0.5
tTile:setLayerZ(layer,z)
18.2.21. tile getLayerZ¶
- getLayerZ(number layer)¶
Get
z
position fromlayer
.- Parameters
number – layer (one based).
- Returns
number
-z
position of layer
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local layer = 1
local z = tTile:getLayerZ(layer)
18.3. tile object¶
Tile Object is an independent object with its physics and own control of render. It inherits from renderizable.
Although it is independent, if the tile map is destroyed, it is also destroyed.
18.3.1. tile setBrickID¶
- setBrickID(number brickID)¶
Set a new Brick ID based on existent in tile map.
- Parameters
number – brick id.
Example:
tTile = tile:new('2dW')
tTile:load('my_map.tile')
local tileID = 144
local layer = 2
tSaw = tTile:createTileObject(tileID,layer)
local brickID = 25
tSaw:setBrickID(brickID)
function loop(delta)
tSaw.az = tSaw.az + math.rad(50)
end
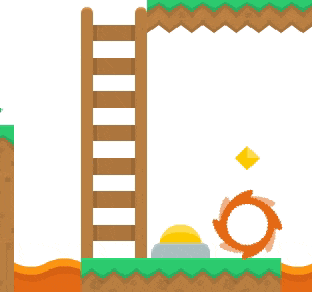
Figure 18.4 Changing the brick ID from Tile Object¶