Table of Contents
5. Table font¶
A table font represent a true type font and can be loaded dynamically or loaded from a binary type generated by the engine.
5.1. font methods¶
5.1.1. font new from true type¶
- new(string file_name, number height, number spaceX, number spaceY, boolean save_image_as_png)¶
Create a new instance of a font passing the file name (extension expected
.ttf
) and the configuration expected.- Parameters
string – file name from true type font
.ttf
.number – height from font in units.
number – spaceX between the axis x.
number – spaceY is the
\n
(new line space).boolean – save_png is a flag that instruct to save the font’s image as png.
- Returns
font table.
Example:
tArial15 = font:new('Arial.ttf',15,0,2,true) --parse runtime a new font
tArial13 = font:new('Arial.ttf',13,0,2,false) --parse runtime other font
Note
5.1.2. font new from a binary file¶
- new(string file_name)¶
Create a new instance of a font passing the binary file name (extension expected
fnt
).- Parameters
string – file name binary. Extension expected
fnt
.
Example:
tArial15 = font:new('Arial15.fnt') -- load a file previously created by the engine.
tArial13 = font:new('Arial13.fnt') -- load a file previously created by the engine.
Note
5.1.3. font setSpace¶
- setSpace(string axis, number value)¶
Change the current space in the axis
x
ory
.- Parameters
string – axis
x
ory
.number – value from space to be applied.
Example:
tArial15 = font:new('Arial.ttf',15,0,2) --parse runtime a new font
tArial15:setSpace('x',18)
tArial15:setSpace('y',0)
5.1.4. font getSpace¶
- getSpace(string axis)¶
Retrieve the current space from font.
- Parameters
string – axis
x
ory
.- Returns
number
- space.
Example:
tArial15 = font:new('Arial.ttf',15,0,2) --parse runtime a new font
local x = tArial15:getSpace('x') -- 0
local y = tArial15:getSpace('y') -- 2
5.1.5. font getHeight¶
tArial15 = font:new('Arial.ttf',15,0,2) --parse runtime a new font
local height = tArial15:getHeight() -- 15
5.1.6. font add¶
- add(string text, string * world, number * x, number * y, number * z)¶
Create a new instance of a text from a font. Same as addText.
- Parameters
string – text to be drew.
string – world can be
2ds
,2dw
or3d
(detail).number – x position (optional).
number – y position (optional).
number – z position (optional).
Example:
tArial15 = font:new('Arial.ttf',15,0,2) --parse runtime a new font
tText = tArial15:add('Hello text!','2ds',100,100)
Note
It might occurs error related to the character encoding. This can be solved changing the text to UTF8 Without BOM
5.1.7. font addText¶
- addText(string text, string * world, number * x, number * y, number * z)¶
Create a new instance of a text from a font. Same as add.
- Parameters
string – text to be drew.
string – world can be
2ds
,2dw
or3d
(detail).number – x position (optional).
number – y position (optional).
number – z position (optional).
Example:
tArial15 = font:new('Arial15.fnt') --load from binary previously generated
tText = tArial15:addText('Hello text!','2dw',200,200)
Note
It might occurs error related to the character encoding. This can be solved changing the text to UTF8 Without BOM
5.2. font methods with editor features¶
Here the methods available when compiled with the flag USE_EDITOR_FEATURES
.
5.2.1. Size of letter¶
- getSizeLetter(string letter)¶
Get the size of specific letter DO NOT considering the rotation neither scale.
- Parameters
string – letter to be retrieved the size
- Returns
number
width,number
height - dimension of letter.
Example:
local height = 20 --the font height
local space_x = 2 --space between characters in points coordinates
local space_y = 1 --space between new line characters in points coordinates
local save_image_as_png = true --will save a local image as 'font_name-20.png'
local base_name = 'Carlito-Regular'
tMyFont = font:new(base_name .. '.ttf',height,space_x,space_y,save_image_as_png)
local width, height = tMyFont:getSizeLetter('A')
print(width,height) -- for this example width:12, height:13
- setSizeLetter(string letter, number width, number height)¶
Force a new size of letter indicating the width and height.
- Parameters
string – letter to be retrieved the size
number – width of letter.
number – height of letter.
Example:
local height = 60 --the font height
local space_x = 2 --space between characters in points coordinates
local space_y = 1 --space between new line characters in points coordinates
local save_image_as_png = true --will save a local image as 'font_name-60.png'
local base_name = 'Carlito-Regular'
tMyFont = font:new(base_name .. '.ttf',height,space_x,space_y,save_image_as_png)
local width, height = tMyFont:getSizeLetter('a')
print(width,height) -- for this example width:12, height:13
tMyFont:setSizeLetter(' ',10,0) --10 it is good space for this font size (I am guessing)
--set the new size of letter
tMyFont:setSizeLetter('a',width * 2, height)
tText = tMyFont:addText("My new size\n Note the 'a'\nsomething stranger",'2dw')
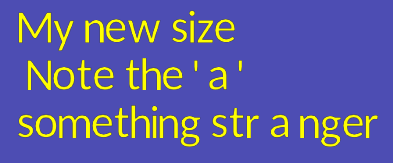
Figure 5.1 Change the dimension of the letter ‘a’¶
5.2.2. Displacement for letter in the axis x, y¶
- getLetterXDiff(string letter)¶
Get the displacement for the specific letter in the axis
X
. By default the value is 0. It can be negative.- Parameters
string – letter to be retrieved the size
- Returns
number
displacement - X of letter.
Example:
local height = 60 --the font height
local space_x = 2 --space between characters in points coordinates
local space_y = 1 --space between new line characters in points coordinates
local save_image_as_png = true --will save a local image as 'font_name-60.png'
local base_name = 'Carlito-Regular'
tMyFont = font:new(base_name .. '.ttf',height,space_x,space_y,save_image_as_png)
tMyFont:setSizeLetter(' ',10,0) --space by default is not good, so we change it
tMyFont:setLetterXDiff('e',10)
local X = tMyFont:getLetterXDiff('e')
print('Displacement:', X)
- setLetterXDiff(string letter, number value)¶
Force a new displacement for the specific letter in the axis
X
. The value is added. It can be negative.- Parameters
string – letter to be retrieved the size
number – value of displacement.
Example:
local height = 60 --the font height
local space_x = 2 --space between characters in points coordinates
local space_y = 1 --space between new line characters in points coordinates
local save_image_as_png = true --will save a local image as 'font_name-60.png'
local base_name = 'Carlito-Regular'
tMyFont = font:new(base_name .. '.ttf',height,space_x,space_y,save_image_as_png)
tMyFont:setSizeLetter(' ',10,0) --space by default is not good, so we change it
tMyFont:setLetterXDiff('e',10)
tText = tMyFont:add('Some Text: Hello World!!!','2dw')

Figure 5.2 Displacement 10 in the axis X
¶
- getLetterYDiff(string letter)¶
Get the displacement for the specific letter in the axis
Y
. By default the value is 0. It can be negative.- Parameters
string – letter to be retrieved the size
- Returns
number
displacement - Y of letter.
Example:
local height = 60 --the font height
local space_x = 2 --space between characters in points coordinates
local space_y = 1 --space between new line characters in points coordinates
local save_image_as_png = true --will save a local image as 'font_name-60.png'
local base_name = 'Carlito-Regular'
tMyFont = font:new(base_name .. '.ttf',height,space_x,space_y,save_image_as_png)
tMyFont:setSizeLetter(' ',10,0) --space by default is not good, so we change it
tMyFont:setLetterYDiff('e',10)
local y = tMyFont:getLetterYDiff('e')
print('Displacement:', y)
- setLetterYDiff(string letter, number value)¶
Force a new displacement for the specific letter in the axis
Y
. The value is added. It can be negative.- Parameters
string – letter to be retrieved the size
number – value of displacement.
Example:
local height = 60 --the font height
local space_x = 2 --space between characters in points coordinates
local space_y = 1 --space between new line characters in points coordinates
local save_image_as_png = true --will save a local image as 'font_name-60.png'
local base_name = 'Carlito-Regular'
tMyFont = font:new(base_name .. '.ttf',height,space_x,space_y,save_image_as_png)
tMyFont:setSizeLetter(' ',10,0) --space by default is not good, so we change it
tMyFont:setLetterYDiff('e',10)
tText = tMyFont:add('Some Text: Hello World!!!','2dw')

Figure 5.3 Displacement 10 in the axis Y
¶
5.3. Creating a font programmatically¶
Here an example how to create a binary font from a true type file format.
For this example it will be used the Carlito-Regular true type font.
Example:
mbm.setColor(0.3,0.3,0.7) --blue color of background
if not mbm.get('USE_EDITOR_FEATURES') then
mbm.messageBox('Missing features','Is necessary to compile using USE_EDITOR FEATURES to run this example','ok','error',0)
mbm.quit()
end
function saveBinaryFont(tText,fileName)
local tMeshDebug = meshDebug:new()
if tMeshDebug:loadFromMemory(tText) then -- require to be compiled using the editor flag: USE_EDITOR_FEATURES
if tMeshDebug:copyAnimationsFromMesh(tText) then --copy animations created
if tMeshDebug:save(fileName) then
print("Sucessfully saved font!")
return true
else
print("Failed to save font!")
end
else
print("Failed to apply shader and animations!")
end
else
print("Failed to load font!")
end
return false
end
function onInitScene()
local height = 50 --the font height
local space_x = 2 --space between characters in points coordinates
local space_y = 1 --space between new line characters in points coordinates
local save_image_as_png = true --will save a local image as 'font_name-50.png'
local base_name = 'Carlito-Regular'
tMyFont = font:new(base_name .. '.ttf',height,space_x,space_y,save_image_as_png)
--add one text to view
local tText = tMyFont:addText('Hello \n Font !\n 0123456789','2dw')
--increase the space width
local xSpace,ySpace = tMyFont:getSizeLetter(' ')
tMyFont:setSizeLetter(' ',10,ySpace) --10 it is good space for this font size (I am guessing)
--add one font color as animation
tText:addAnim('red font animation')
--get the shader of font to change it
local shader = tText:getShader()
shader:load('font.ps') --just pixel shader, vertex shader is not necessary
shader:setPSall('colorFont',1,0,0) --change color font to red for min, max and current
local fileNameBinary = base_name .. '.fnt'
if saveBinaryFont(tText,fileNameBinary) then
tText:destroy() --destroy the existing one to reload from binary file previously created
tMyFont = font:new(fileNameBinary) --load from binary font
tText = tMyFont:addText('Hello world \n Font !\n 0123456789 \n #2# Second Animation','2dw')
tText:setWildCard('#')--instruct to look a number between '#' that indicate the animation
end
end
download font_creating_example_1.lua
.
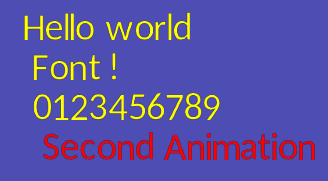
Figure 5.4 font_creating_example_1.lua running¶
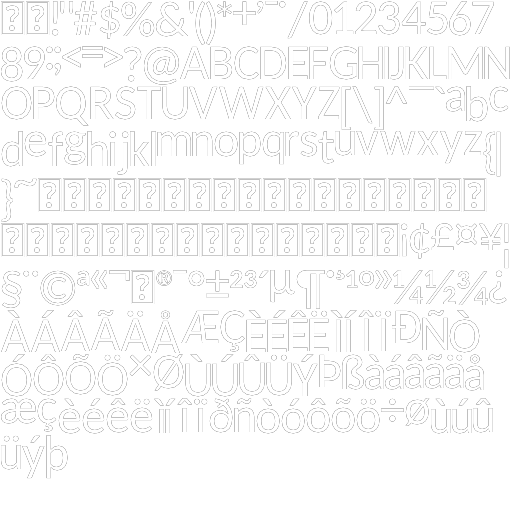
Figure 5.5 Carlito-Regular png generated¶