Table of Contents
7. Table line¶
A table line inherit from renderizable and implement all methods as usual. Also line has a default animation with a particular shader table which can be accessed by getShader method as usual.
This table allow to create lines dynamically. Each table line might have one or more lines.
7.1. line methods¶
7.1.1. line new¶
- new(string * world, number * x, number * y, number * z)¶
Create a new instance of a line passing the world desired (detail) and position.
- Parameters
string – world can be
2ds
,2dw
or3d
.number – x position (optional).
number – y position (optional).
number – z position (optional).
- Returns
line table.
Example:
tLine = line:new('2dw')
7.1.2. line add¶
- add(table[x,y,*z])¶
Add new line. Optionally the table might have
z
coordinate. Eachx,y,*z
is a point in the line.- Parameters
table – points to line.
- Returns
number
- index from current line (1 based).
Example:
tLine = line:new('2dw')
local tPoints = {0,0, 100,100} --minimum two points to be a line
local index = tLine:add(tPoints)
print('Line added index:',index)
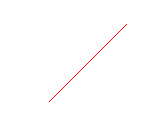
Figure 7.1 Draw a simple line at 2d world.¶

Figure 7.2 Output.¶
Note the difference to 2D screen. more detail
tLine = line:new('2ds')-- 2d screen
local tPoints = {0,0, 100,100} --minimum two points to be a line
local index = tLine:add(tPoints)

7.1.3. line set¶
- set(table[x,y,*z], number index)¶
Update the line passing the table and index (1 based).
- Parameters
table – points to line.
number – index to line.
Example:
tLine = line:new('2ds')
local t = {0,0, 0,500, 500,500, 500, 0, 0,0 }
local index = tLine:add(t)
local tNewLine = {0,0, 0,250, 250,250, 250, 0, 0,0 }
tLine:set(tNewLine,index)
7.1.4. line setColor¶
- setColor(number r, number g, number b, number * a)¶
Update the color from line passing the color RGB and optionally alpha.
- Parameters
number – r red color (0 to 1).
number – g green color (0 to 1).
number – b blue color (0 to 1).
number – a alpha color (0 to 1) (optional).
Example:
mbm.setColor(0,0,0) --set background color to black
tLine = line:new('2dw')
local tXY = {-50,-50, -50,50, 50,50, 50,-50, -50,-50 }
local index = tLine:add(tXY)
tLine:setColor(1,1,1) --white color
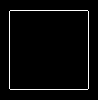
Figure 7.3 Set line color to white¶
7.1.5. line size¶
- size¶
Retrieve the size of lines added.
- Returns
number
- size of lines added.
Example:
tLine = line:new('2ds')
local t = {0,0, 0,500, 500,500, 500, 0, 0,0 }
local index = tLine:add(t)
local size = tLine:size() -- 1
7.1.6. line drawBounding¶
- drawBounding(table renderizable, boolean * useAABB)¶
Draw a bounding from a renderizable. The flag useAABB consider the rotation from a object.
- Parameters
renderizable – render to set values from physics.
boolean – useAABB is a flag enable AABB (default
true
).
Example:
mbm.setColor(0,0,0) --set background color to black
tTexture = texture:new('2dw') -- new texture
tTexture:load('#ffffffff') -- load white color
tTexture:setSize(150,50)
tLine = line:new('2dw')
tLine:drawBounding(tTexture)
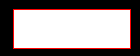
Figure 7.4 Draw a simple bounding line. Get the bound from texture.¶
Disabled AABB:
mbm.setColor(0,0,0) --set background color to black
tTexture = texture:new('2dw') -- new texture
tTexture:load('#ffffffff') -- load white color
tTexture:setSize(150,50)
tLine = line:new('2dw')
tLine:drawBounding(tTexture)

Figure 7.5 Draw a simple bounding line. Get the size from texture not considering AABB.¶
Enabled AABB:
mbm.setColor(0,0,0) --set background color to black
tTexture = texture:new('2dw') -- new texture
tTexture:load('#ffffffff') -- load white color
tTexture:setSize(150,50)
tLine = line:new('2dw')
tLine:drawBounding(tTexture,true)
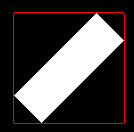
Figure 7.6 Draw a simple bounding line. Get the size from texture considering AABB.¶
7.1.7. line setPhysics¶
- setPhysics(table renderizable, boolean * bDrawLines)¶
Set physic from a renderizable (copy the values to itself). The flag
bDrawLines
instruct to draw the physics line. It is used in general for debugging proposal.- Parameters
renderizable – render to set values from physics.
boolean – bDrawLines instruct to draw the line.
Example:
tShape = shape:new('2dw')
tShape:create('rectangle',100,50)
local bDrawLines = true
tLine = line:new('2dw')
tLine:setPhysics(tShape,bDrawLines)
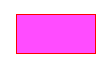
Figure 7.7 Setting the physics and drawing it.¶
7.1.8. line setPhysics¶
- setPhysics(table tPhysics, boolean * bDrawLines)¶
- Apply physics from a table. The flag
bDrawLines
instruct to draw the physics line.It is very useful in general for debugging proposal.- Parameters
table – info to set values to physics.
boolean – bDrawLines instruct to draw the line (default is
false
).
The following table describe the table available for the primitives 2d types:
type /nameposition x,yinformation ?width, heightinformation ?pointsinformation ?rayinformation ?rect
yes
yes
no
no
circle
yes
yes
no
yes
ellipse
yes
yes
no
yes
triangle
yes
no
yes
no
polygon
yes
no
yes
no
Example:
1 function onInitScene() 2 3 mbm.setColor(0,0,0) --set background color to black 4 5 local bDrawLines = true --instruct to draw the lines 6 local tRect = {type = 'rect', width=100, height=100, x=0, y=0} 7 local tCircle = {type = 'circle', ray = 50, x=0, y=0} 8 local tEllipse = {type = 'ellipse', width=100, height=70, x=0, y=0} 9 local tTriangle = {type = 'triangle', x=0, y=0, points={[1]={x=-50,y=-50},--must have 3 points 10 [2]={x=0,y=50}, 11 [3]={x=50,y=-50}}} 12 local tPolygon = {type = 'polygon', x=0, y=0, points={ --each 3 points 13 [1]={x=-50,y=-50}, 14 [2]={x=0,y=0}, 15 [3]={x=40,y=-40}, 16 [4]={x=50,y=50}, 17 [5]={x=0,y=0}, 18 [6]={x=-50,y=50}, 19 [7]={x=-250,y=250},--this point will be ignored 20 }} 21 22 23 tLineRect = line:new('2dw') 24 tLineRect:setPhysics(tRect,bDrawLines) 25 tLineRect.x = -200 26 27 tLineCircle = line:new('2dw') 28 tLineCircle:setPhysics(tCircle,bDrawLines) 29 tLineCircle.x = -100 30 31 tLineEllipse = line:new('2dw') 32 tLineEllipse:setPhysics(tEllipse,bDrawLines) 33 tLineEllipse.x = 0 34 35 tLineTriangle = line:new('2dw') 36 tLineTriangle:setPhysics(tTriangle,bDrawLines) 37 tLineTriangle.x = 100 38 39 tLinePolygon = line:new('2dw') 40 tLinePolygon:setPhysics(tPolygon,bDrawLines) 41 tLinePolygon.x = 200 42 43 tAllObjs = {} 44 table.insert(tAllObjs,tLineRect) 45 table.insert(tAllObjs,tLineCircle) 46 table.insert(tAllObjs,tLineEllipse) 47 table.insert(tAllObjs,tLineTriangle) 48 table.insert(tAllObjs,tLinePolygon) 49 50 end 51 52 53 function loop(delta) 54 for i=1, #tAllObjs do 55 local tObj = tAllObjs[i] 56 tObj:rotate('z',math.rad(90)) 57 end 58 end
Figure 7.8 Example creating physics from lua table.¶
7.2. line shader¶
The equivalent using table shader is:
mbm.setColor(0,0,0)
--common
local r,g,b,a = 1,1,1,1
local tXY = {-50,-50, -50,50, 50,50, 50,-50, -50,-50 }
--using setColor to modify the line color
tLine_1 = line:new('2dw')
local index = tLine_1:add(tXY)
tLine_1:setColor(r,g,b,a) --set the variable color in the shader to white
--using shader to modify the line color
tLine_2 = line:new('2dw',200)--200 to right (x)
index = tLine_2:add(tXY)
local tShader = tLine_2:getShader()
tShader:setPSall('color',r,g,b,a)--set the variable color in the shader to white
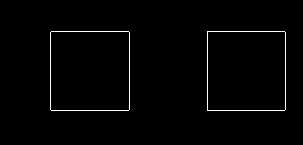
Figure 7.9 changing color using setcolor and shader directly.¶
7.2.1. line fragment shader¶
The fragment shader (pixel shader) used for the line is:
precision mediump float;
uniform vec4 color;
void main()
{
gl_FragColor = color;
}
7.2.2. line vertex shader¶
The vertex shader used for the line is:
attribute vec4 aPosition;
uniform mat4 mvpMatrix;
void main()
{
gl_Position = mvpMatrix * aPosition;
}