Table of Contents
13. Table shader¶
A table shader is responsible for the effect shader in the renderizable table. Each animation has an effect shader which can be modified by the shader methods and also it has its own animation.
Use getShader to retrieve a shader table.
Use getShaderList to get shader information.
All shader code are described at shader code.
13.1. shader methods¶
13.1.1. shader load¶
- load(string pixelFileName, string vertexFileName, *number typeAnimPs, *number timeAnimPs, *number typeAnimVs, *number timeAnimVs)¶
Load a new shader supplying arguments needed.
- Parameters
string – pixelFileName must exists or can be nil.
string – vertexFileName can be nil (optional) .
number – typeAnimPs Type animation constant for pixel shader. (optional, default is
GROWING
), see type of animationnumber – timeAnimPs Animation time for pixel shader. (optional, default is 0.0).
number – typeAnimVs Type animation constant for vertex shader. (optional, default is
GROWING
), see type of animationnumber – timeAnimVs Animation time for vertex shader. (optional, default is 0.0).
- Returns
boolean
- result
Example
myMesh1 = mesh:new('2dw',-100)-- create one object as 2d in the left (x:-100)
myMesh2 = mesh:new('2dw', 100)-- create second object as 2d in the right (x:100)
if myMesh1:load('crate.msh') then
print("crate 1 successfully loaded " )
else
print('Failed to load crate.msh (1)')
end
if myMesh2:load('crate.msh') then
print("crate 2 successfully loaded ... let's apply a shader to it" )
local tShader = myMesh2:getShader()
if tShader:load('brightness.ps',nil,mbm.GROWING,1.0,mbm.GROWING_LOOP,2.0) then
print('Successfully loaded shader ')
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh (2)')
end
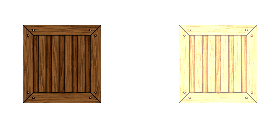
Figure 13.1 Applying shader and compare to an object without shader¶
download crate.msh
download crate.png
Note
nil
if not applicable.13.1.2. shader setPS¶
13.1.2.1. shader setPS¶
- setPS(string name, number value_1, ...)¶
Set new value to a variable ‘current’ into pixel shader passing the variable name and values (one or more, depends on type).
- Parameters
string – name variable.
number – value of variable (can be one or more, depends on shader variable).
Example:
mbm.setColor(0,0,0) --background black
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color it.ps',nil,mbm.PAUSED,1.0,mbm.PAUSED,0.0) then
local r,g,b = 0,1,0 --rgb green color
tShader:setPS('color',r,g,b) --set green color to current shader
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
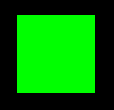
Figure 13.2 Change the current value from a variable in the current shader¶
13.1.2.2. shader setPSmin¶
- setPSmin(string name, number value_1, ...)¶
Set the minimum value to a variable into pixel shader passing the variable name and values (one or more, depends on type).
- Parameters
string – name variable.
number – value of variable (can be one or more, depends on shader variable).
Example:
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color it.ps',nil,mbm.GROWING_LOOP,0.5,mbm.PAUSED,0.0) then
local min_r,min_g,min_b = 0,0,0 --rgb black color
local max_r,max_g,max_b = 1,0,0 --rgb red color
tShader:setPSmin('color',min_r,min_g,min_b) --set black color as min color
tShader:setPSmax('color',max_r,max_g,max_b) --set red color as max color
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
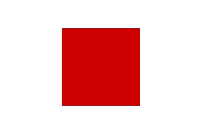
Figure 13.3 Change the min value from a variable in the current shader¶
13.1.2.3. shader setPSmax¶
- setPSmax(string name, number value_1, ...)¶
Set the maximum value to a variable into pixel shader passing the variable name and values (one or more, depends on type).
- Parameters
string – name variable.
number – value of variable (can be one or more, depends on shader variable).
Example:
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color it.ps',nil,mbm.GROWING_LOOP,0.5,mbm.PAUSED,0.0) then
local min_r,min_g,min_b = 0,0,0 --rgb black color
local max_r,max_g,max_b = 1,1,1 --rgb white color
tShader:setPSmin('color',min_r,min_g,min_b) --set black color as min color
tShader:setPSmax('color',max_r,max_g,max_b) --set white color as max color
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
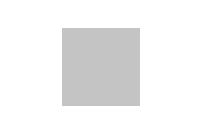
Figure 13.4 Change the max value from a variable in the current shader¶
13.1.2.4. shader setPSall¶
- setPSall(string name, number value_1, ...)¶
Set all values (minimum, maximum and current) to a variable into pixel shader passing the variable name and values (one or more, depends on type).
- Parameters
string – name variable.
number – value of variable (can be one or more, depends on shader variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('brightness.ps',nil,mbm.GROWING,0.0,mbm.PAUSED,0.0) then
local contrast_value = 2
local brightness_value = 0.7
tShader:setPSall('contrast',contrast_value) --set all min, max and current to contrast_value
tShader:setPSall('brightness',brightness_value) --set all min, max and current to brightness_value
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end

Figure 13.5 Change the min/max and current value from a variable in the current shader¶
13.1.2.5. shader setPStime¶
- setPStime(number seconds)¶
Change the time (duration) for the current shader.
- Parameters
number – time in seconds.
Example:
mbm.setColor(1,1,1) -- background white
myMesh1 = mesh:new('2dw',-100) --left
if myMesh1:load('crate.msh') then
local tShader = myMesh1:getShader()
if tShader:load('brightness.ps',nil,mbm.RECURSIVE_LOOP,1.0,mbm.PAUSED,0.0) then
tShader:setPStime(3) --set to 3 second
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
myMesh2 = mesh:new('2dw',100) --right
if myMesh2:load('crate.msh') then
local tShader = myMesh2:getShader()
if tShader:load('brightness.ps',nil,mbm.RECURSIVE_LOOP,1.0,mbm.PAUSED,0.0) then
tShader:setPStime(0.5) --set to 0.5 second
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
13.1.2.6. shader setPStype¶
- setPStype(number type)¶
Change the type of animation for the current shader.
- Parameters
number – type of animation.
Example:
mbm.setColor(1,1,1) -- background white
myMesh1 = mesh:new('2dw',-100) --left
if myMesh1:load('crate.msh') then
local tShader = myMesh1:getShader()
if tShader:load('brightness.ps',nil,mbm.RECURSIVE_LOOP,1.0,mbm.PAUSED,0.0) then
tShader:setPStype(mbm.GROWING_LOOP) --set type to GROWING_LOOP
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
myMesh2 = mesh:new('2dw',100) --right
if myMesh2:load('crate.msh') then
local tShader = myMesh2:getShader()
if tShader:load('brightness.ps',nil,mbm.RECURSIVE_LOOP,1.0,mbm.PAUSED,0.0) then
tShader:setPStype(mbm.DECREASING_LOOP) --set type to DECREASING_LOOP
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
13.1.3. shader setVS¶
13.1.3.1. shader setVS¶
- setVS(string name, number value_1, ...)¶
Set new value to a variable into vertex shader passing the variable name and values (one or more, depends on type).
- Parameters
string – name variable.
number – value of variable (can be one or more, depends on shader variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load(nil,'scale.vs',mbm.PAUSED,0.0,mbm.PAUSED,0.0) then
local sx,sy,sz = 2,1,1 --stretch on x axis
tShader:setVS('scale',sx,sy,sz) --set the current scale
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh (1)')
end
13.1.3.2. shader setVSmin¶
- setVSmin(string name, number value_1, ...)¶
Set the minimum value to a variable into vertex shader passing the variable name and values (one or more, depends on type).
- Parameters
string – name variable.
number – value of variable (can be one or more, depends on shader variable).
Example:
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load(nil,'scale.vs',mbm.PAUSED,0.0,mbm.RECURSIVE_LOOP,1.0) then
local min_x,min_y,min_z = 1,1,1
local max_x,max_y,max_z = 2,2,2
tShader:setVSmin('scale',min_x,min_y,min_z) --set min scale
tShader:setVSmax('scale',max_x,max_y,max_z) --set max scale
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh (1)')
end
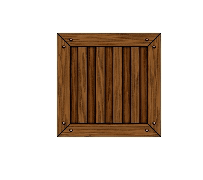
Figure 13.9 Applying min value to vertex shader¶
13.1.3.3. shader setVSmax¶
- setVSmax(string name, number value_1, ...)¶
Set the maximum value to a variable into vertex shader passing the variable name and values (one or more, depends on type).
- Parameters
string – name variable.
number – value of variable (can be one or more, depends on shader variable).
Example:
*Example:*
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load(nil,'scale.vs',mbm.PAUSED,0.0,mbm.RECURSIVE_LOOP,1.0) then
local min_x,min_y,min_z = 1,1,1
local max_x,max_y,max_z = 2,2,2
tShader:setVSmin('scale',min_x,min_y,min_z) --set min scale
tShader:setVSmax('scale',max_x,max_y,max_z) --set max scale
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh (1)')
end
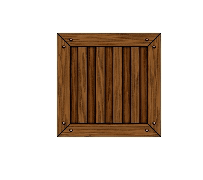
Figure 13.10 Applying max value to vertex shader¶
13.1.3.4. shader setVSall¶
- setVSall(string name, number value_1, ...)¶
Set all values (minimum, maximum and current) to a variable into vertex shader passing the variable name and values (one or more, depends on type).
- Parameters
string – name variable.
number – value of variable (can be one or more, depends on shader variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load(nil,'scale.vs',mbm.GROWING,0.0,mbm.PAUSED,0.0) then
local sx,sy,sz = 2,0.5,1 --stretch on x axis and shrink it in the y axis
tShader:setVSall('scale',sx,sy,sz) --set the current scale
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh (1)')
end
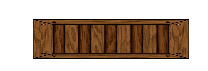
Figure 13.11 Set all values (min, max and current) to a variable into a vertex shader¶
13.1.3.5. shader setVStime¶
- setVStime(number seconds)¶
Change the time (duration) for the current shader.
- Parameters
number – time in seconds.
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load(nil,'scale.vs',mbm.PAUSED,0.0,mbm.RECURSIVE_LOOP,1.0) then
local min_x,min_y,min_z = 1,1,1
local max_x,max_y,max_z = 2,2,2
tShader:setVSmin('scale',min_x,min_y,min_z)
tShader:setVSmax('scale',max_x,max_y,max_z)
tShader:setVStime(0.2)
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh (1)')
end
13.1.3.6. shader setVStype¶
- setVStype(number type)¶
Change the type of animation for the current shader.
- Parameters
number – type of animation.
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load(nil,'scale.vs',mbm.PAUSED,0.0,mbm.RECURSIVE_LOOP,1.0) then
local min_x,min_y,min_z = 1,1,1
local max_x,max_y,max_z = 2,2,2
tShader:setVSmin('scale',min_x,min_y,min_z)
tShader:setVSmax('scale',max_x,max_y,max_z)
tShader:getVS(0.2)
tShader:setVStype(mbm.GROWING_LOOP)
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh (1)')
end
13.1.4. shader getPS¶
13.1.4.1. shader getPS¶
- getPS(string name)¶
Get the current variable’s value from pixel shader passing the variable name.
- Parameters
string – name of variable.
- Returns
number
value of variable (one or more value, depends on type of variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color tone.ps',nil,mbm.GROWING,0.0,mbm.PAUSED,0.0) then
local desaturation = tShader:getPS('desaturation')
local toned = tShader:getPS('toned')
local lightColor_r,lightColor_g,lightColor_b,lightColor_a = tShader:getPS('lightColor')
local darkColor_r,darkColor_g,darkColor_b,darkColor_a = tShader:getPS('darkColor')
print('desaturation',desaturation)
print('toned', toned)
print('lightColor',lightColor_r,lightColor_g,lightColor_b,lightColor_a)
print('darkColor ',darkColor_r, darkColor_g, darkColor_b, darkColor_a )
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
13.1.4.2. shader getPSmin¶
- getPSmin(string name)¶
Get the minimum variable’s value from pixel shader passing the variable name.
- Parameters
string – name of variable.
- Returns
number
value of variable (one or more value, depends on type of variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color tone.ps',nil,mbm.GROWING,0.0,mbm.PAUSED,0.0) then
local desaturation = tShader:getPSmin('desaturation')
local toned = tShader:getPSmin('toned')
local lightColor_r,lightColor_g,lightColor_b,lightColor_a = tShader:getPSmin('lightColor')
local darkColor_r,darkColor_g,darkColor_b,darkColor_a = tShader:getPSmin('darkColor')
print('min desaturation',desaturation)
print('min toned', toned)
print('min lightColor',lightColor_r,lightColor_g,lightColor_b,lightColor_a)
print('min darkColor ',darkColor_r, darkColor_g, darkColor_b, darkColor_a )
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
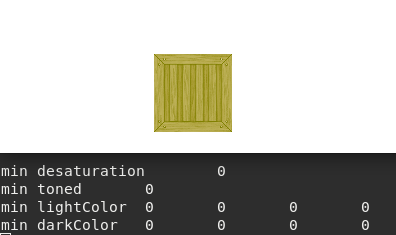
Figure 13.15 getting minimum value of variable in pixel shader¶
13.1.4.3. shader getPSmax¶
- getPSmax(string name)¶
Get the maximum variable’s value from pixel shader passing the variable name.
- Parameters
string – name of variable.
- Returns
number
value of variable (one or more value, depends on type of variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color tone.ps',nil,mbm.GROWING,0.0,mbm.PAUSED,0.0) then
local desaturation = tShader:getPSmax('desaturation')
local toned = tShader:getPSmax('toned')
local lightColor_r,lightColor_g,lightColor_b,lightColor_a = tShader:getPSmax('lightColor')
local darkColor_r,darkColor_g,darkColor_b,darkColor_a = tShader:getPSmax('darkColor')
print('max desaturation',desaturation)
print('max toned', toned)
print('max lightColor',lightColor_r,lightColor_g,lightColor_b,lightColor_a)
print('max darkColor ',darkColor_r, darkColor_g, darkColor_b, darkColor_a )
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
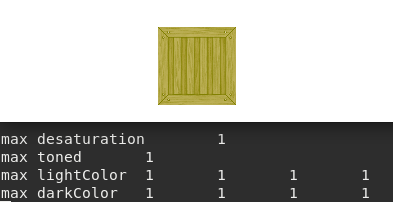
Figure 13.16 getting maximum value of variable in pixel shader¶
13.1.4.4. shader getPStime¶
- getPStime¶
Get the time effect from pixel shader passing the variable name.
- Returns
number
time in seconds of the effect.
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color tone.ps',nil,mbm.GROWING,0.0,mbm.PAUSED,0.0) then
local my_mtime = tShader:getPStime()
print('time',my_mtime)
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
13.1.4.5. shader getPStype¶
- getPStype¶
Get the type animation from pixel shader passing the variable name.
- Returns
- literal
type of animation as
string
,type
as number.
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color tone.ps',nil,mbm.GROWING,0.0,mbm.PAUSED,0.0) then
local sType,iType = tShader:getPStype()
print('type as string :' .. sType, 'type as integer:', iType)
--output: type as string :GROWING type as integer: 1
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
13.1.5. shader getVS¶
13.1.5.1. shader getVS¶
- getVS(string name)¶
Get the current variable’s value from vertex shader passing the variable name.
- Parameters
string – name of variable.
- Returns
number
value of variable (one or more value, depends on type of variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color tone.ps','scale.vs',mbm.GROWING,0.0,mbm.PAUSED,0.0) then
tShader:setVS('scale',2,2,2)
local xScale,yScale,zScale = tShader:getVS('scale')
print('The current value of scale is:',xScale,yScale,zScale)
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
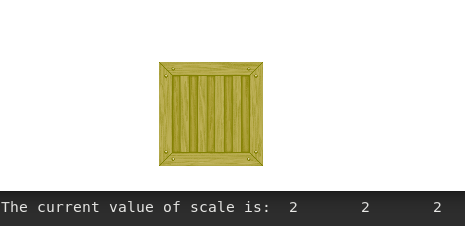
Figure 13.17 getting the value of variable from vertex shader¶
13.1.5.2. shader getVSmin¶
- getVSmin(string name)¶
Get the minimum variable’s value from vertex shader passing the variable name.
- Parameters
string – name of variable.
- Returns
number
value of variable (one or more value, depends on type of variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color tone.ps','scale.vs',mbm.GROWING,0.0,mbm.PAUSED,0.0) then
tShader:setVS('scale',2,2,2)
local x_min,y_min,z_min = tShader:getVSmin('scale')
print('The minimum value of scale is:',x_min,y_min,z_min)
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
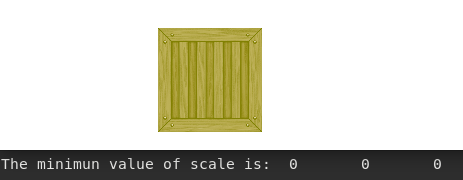
Figure 13.18 getting the minimum value of variable from vertex shader¶
13.1.5.3. shader getVSmax¶
- getVSmax(string name)¶
Get the maximum variable’s value from vertex shader passing the variable name.
- Parameters
string – name of variable.
- Returns
number
value of variable (one or more value, depends on type of variable).
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load('color tone.ps','scale.vs',mbm.GROWING,0.0,mbm.PAUSED,0.0) then
tShader:setVS('scale',2,2,2)
local x_max,y_max,z_max = tShader:getVSmax('scale')
print('The maximum value of scale is:',x_max,y_max,z_max)
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
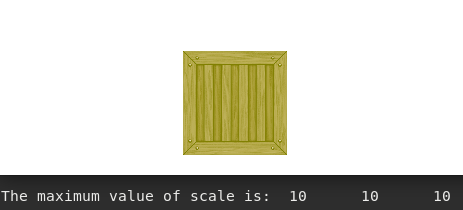
Figure 13.19 getting the maximum value of variable from vertex shader¶
13.1.5.4. shader getVStime¶
- getVStime¶
Get the time effect from vertex shader passing the variable name.
- Returns
number
time in seconds of the effect.
Example:
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load(nil,'scale.vs',mbm.PAUSED,0.0,mbm.GROWING,0.0) then
local i_time = tShader:getVStime()
print('time:',i_time)
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
13.1.5.5. shader getVStype¶
- getVStype¶
Get the type animation from vertex shader passing the variable name.
- Returns
- literal
type of animation as
string
,type
as number.
Example:
mbm.setColor(1,1,1) -- background white
myMesh = mesh:new('2dw')
if myMesh:load('crate.msh') then
local tShader = myMesh:getShader()
if tShader:load(nil,'scale.vs',mbm.PAUSED,0.0,mbm.GROWING,0.0) then
local sType,iType = tShader:getVStype()
print('type as string :' .. sType, 'type as integer:', iType)
--output: type as string :GROWING type as integer: 1
else
print('Failed to load shader ...')
end
else
print('Failed to load crate.msh')
end
13.1.6. shader util¶
The following methods are useful mostly for editors.
13.1.6.1. shader getNames¶
- getNames¶
Retrieve the names (pixel and vertex) from current shader.
- Returns
string
pixel shader name,string
vertex shader name (might returnnil
)
13.1.6.2. shader getCode¶
- getCode¶
Retrieve the code (pixel and vertex) from current shader.
- Returns
string
pixel shader code,string
vertex shader code (might returnnil
)
13.1.6.3. shader getVars¶
- getVars¶
Retrieve an array of table with information (pixel and vertex) from current shader for each variable.
- Returns
table
pixel shader variables,table
vertex shader variables
The table will have the following structure:
Field
Expected as
Detail type
name
string
variable name
type
number
vec2
vec3
rgb
rgba
{[1] = value}
{[1] = x,[2] = y}
{[1] = x,[2] = y, [3] = z}
{[1] = r,[2] = g, [3] = b}
{[1] = r,[2] = g, [3] = b, [4] = a}
value
min
max
Example:
{ [1] = { name = 'color',
type = 'rgb',
value = {[1] = r, [2] = g, [3] = b},
min = {[1] = r, [2] = g, [3] = b},
max = {[1] = r, [2] = g, [3] = b} }, [2] = {...} }
13.1.6.4. shader getTextureStage2¶
- getTextureStage2¶
Retrieve the current texture applied to stage 2 (if there is) (might return
nil
)- Returns
string
texture file name
13.1.7. shader setBlendOp¶
Blend state or Blending is the stage of OpenGL rendering pipeline that takes the fragment color outputs from the Fragment Shader and combines them with the colors in the color buffers that these outputs map to.
Blending parameters can allow the source and destination colors for each output to be combined in various ways.
The color
S
is the source color; the colorD
is the destination color; the colorO
is the output color that is written to the buffer.The
S
,D
, and so forth represent all of the components of that color.
Srgb
represents only theRGB
components of the source color. Da represents the alpha component of the destination color.You can learn more at wiki Blending.
Function
Explanation
ADD
The source and destination colors are added to each other.O = sS + dD
.Thes
andd
are blending parameters that are multiplied into each ofS
andD
before the addition.SUBTRACT
Subtracts the destination from the source.O = sS - dD
.The source and dest are multiplied by blending parameters.REVERSE_SUBTRACT
Subtracts the source from the destination.O = dD - sS
.The source and dest are multiplied by blending parameters.MIN
The output color is the component-wise minimum value of the source and dest colors.So performingGL_MIN
in theRGB
equation means thatOr = min(Sr, Dr), Og = min(Sg, Dg)
, and so forth.The parameters s and d are ignored for this equation.MAX
The output color is the component-wise maximum value of the source and dest colors.The parameterss
andd
are ignored for this equation.
Blend operation is also described at blend state topic.
- setBlendOp(string name)¶
Set the blend operation to a renderizable.
- Parameters
string – name regard the :blend operation.
Example:
local tShape = shape:new('2DW')
tShape:create('circle')
local tShader = tShape:getShader()
tShader:setBlendOp('MIN')
- setBlendOp(number constant)¶
Set the blend operation to a renderizable.
- Parameters
string – name regard the :blend operation.
Example:
local tShape = shape:new('2DW')
tShape:create('circle')
local tShader = tShape:getShader()
tShader:setBlendOp(mbm.MAX)
13.1.8. shader getBlendOp¶
- getBlendOp¶
Get the blend operation from a renderizable.
- Returns
string
name - blend operation of renderizable.
Example:
local tShape = shape:new('2DW')
tShape:create('circle')
local tShader = tShape:getShader()
print(tShader:getBlendOp()) -- expected 'ADD'
13.2. pixel shader¶
Bellow all embedded pixel shader available for the engine:
13.2.1. bands¶
- name
bands.ps
- variable
bandIntensity
- min
0.001
- max
0.56
- default
0.56
- variable
bandDensity
- min
0
- max
150
- default
65
- shader code
precision mediump float;
uniform float bandDensity;
uniform float bandIntensity;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec4 color)
{
color.xyz += (tan((vTexCoord.x * bandDensity)) * bandIntensity);
return color;
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
gl_FragColor = xlat_main(color);
}
13.2.2. blend¶
- name
blend.ps
- variable
junctionRemove
- min
0, 0, 0, 0
- max
1, 1, 1, 1
- default
0.2, 0.2, 0.2, 0
- variable
colorAdd
- min
0, 0, 0
- max
1, 1, 1
- default
1, 0, 0
- variable
invertSample
- min
0
- max
1
- default
0
- variable
disableSample1
- min
0
- max
1
- default
0
- shader code
precision mediump float;
uniform vec3 colorAdd;
uniform vec4 junctionRemove;
uniform sampler2D sample0;
uniform sampler2D sample1;
uniform float invertSample;
uniform float disableSample1;
varying vec2 vTexCoord;
void main()
{
vec4 c0;
vec4 c1;
vec4 original;
vec4 outColor;
if (invertSample > 0.5)
{
c0 = texture2D(sample1, vTexCoord.xy);//sample1 precisa ter alpha
c1 = texture2D(sample0, vTexCoord.xy);//sample0 nao precisa ter alpha
}
else
{
c0 = texture2D(sample0, vTexCoord.xy);//sample0 nao precisa ter alpha
c1 = texture2D(sample1, vTexCoord.xy);//sample1 precisa ter alpha
}
if(disableSample1 > 0.5)
{
c0.rgb += colorAdd;
gl_FragColor = c0;
}
else
{
c1 -= junctionRemove;
original = c0;
outColor.w = c0.w ;
c1.xyz = (c1.xyz * c0.w );
outColor.xyz = ((c0.xyz * (1.0 - c1.w )) + c1.xyz );
outColor = mix( original, outColor, vec4( c1.w ));
outColor.xyz += colorAdd;
gl_FragColor = outColor;
}
}
13.2.3. bloom¶
- name
bloom.ps
- variable
BloomIntensity
- min
0
- max
2
- default
1
- variable
BaseIntensity
- min
0
- max
2
- default
0.5
- variable
BaseSaturation
- min
0
- max
2
- default
0.5
- variable
BloomSaturation
- min
0
- max
2
- default
1
- shader code
precision mediump float;
uniform float BaseIntensity;
uniform float BaseSaturation;
uniform float BloomIntensity;
uniform float BloomSaturation;
uniform sampler2D sample0;
varying vec2 vTexCoord;
float xlat_lib_saturate(float x)
{
return clamp(x, 0.0, 1.0);
}
vec2 xlat_lib_saturate(vec2 x)
{
return clamp(x, 0.0, 1.0);
}
vec3 xlat_lib_saturate(vec3 x)
{
return clamp(x, 0.0, 1.0);
}
vec4 xlat_lib_saturate(vec4 x)
{
return clamp(x, 0.0, 1.0);
}
mat2 xlat_lib_saturate(mat2 m)
{
return mat2(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0));
}
mat3 xlat_lib_saturate(mat3 m)
{
return mat3(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0));
}
mat4 xlat_lib_saturate(mat4 m)
{
return mat4(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0), clamp(m[3], 0.0, 1.0));
}
vec3 AdjustSaturation(in vec3 color, in float saturation)
{
float grey;
grey = dot(color, vec3(0.3, 0.59, 0.11));
return vec3(mix(grey, float(color.xyz), saturation));
}
vec4 xlat_main(in vec4 color)
{
float BloomThreshold = 0.250000;
vec3 base;
vec3 bloom;
base = (color.xyz / color.w);
bloom = xlat_lib_saturate(((base - BloomThreshold) / (1.00000 - BloomThreshold)));
bloom = (AdjustSaturation(bloom, BloomSaturation) * BloomIntensity);
base = (AdjustSaturation(base, BaseSaturation) * BaseIntensity);
base *= (1.00000 - xlat_lib_saturate(bloom));
return vec4(((base + bloom) * color.w), color.w);
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
if(color.a == 0.0)
discard;
else
gl_FragColor = xlat_main(color);
}
13.2.4. blur directional¶
- name
blur directional.ps
- variable
blurAmount
- min
0
- max
0.01
- default
0
- variable
angle
- min
0
- max
360
- default
0
- shader code
precision mediump float;
uniform float angle;
uniform float blurAmount;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv,vec4 color)
{
vec4 c;
float rad;
float xOffset;
float yOffset;
int i = 0;
c = vec4(0.0);
rad = (angle * 0.0174533);
xOffset = cos(rad);
yOffset = sin(rad);
for (; (i < 16); (++i))
{
uv.x = (uv.x - (blurAmount * xOffset));
uv.y = (uv.y - (blurAmount * yOffset));
c += texture2D(sample0, uv);
}
c /= 16.0000;
return c;
}
void main()
{
vec4 color = texture2D(sample0, vTexCoord);
gl_FragColor = xlat_main(vTexCoord,color);
}
13.2.5. blur zoom¶
- name
blur zoom.ps
- variable
center
- min
0, 0
- max
1, 1
- default
0.62, 0.67
- variable
blurAmount
- min
0
- max
2
- default
0.2
- shader code
precision mediump float;
uniform float blurAmount;
uniform vec2 center;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv)
{
vec4 color;
vec4 c;
int i = 0;
float scale;
c = vec4(0.000000);
uv -= center;
for (; (i < 15); (++i))
{
scale = (1.00000 + (blurAmount * (float(i) / 14.0000)));
c += texture2D(sample0, ((uv * scale) + center));
}
c /= 15.0000;
return c;
}
void main()
{
vec4 color = texture2D(sample0, vTexCoord);
gl_FragColor = xlat_main(vTexCoord);
}
13.2.6. bright extract¶
- name
bright extract.ps
- variable
threshold
- min
0
- max
1
- default
0.5
- shader code
precision mediump float;
uniform sampler2D sample0;
uniform float threshold;
varying vec2 vTexCoord;
float xlat_lib_saturate(float x)
{
return clamp(x, 0.0, 1.0);
}
vec2 xlat_lib_saturate(vec2 x)
{
return clamp(x, 0.0, 1.0);
}
vec3 xlat_lib_saturate(vec3 x)
{
return clamp(x, 0.0, 1.0);
}
vec4 xlat_lib_saturate(vec4 x)
{
return clamp(x, 0.0, 1.0);
}
mat2 xlat_lib_saturate(mat2 m)
{
return mat2(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0));
}
mat3 xlat_lib_saturate(mat3 m)
{
return mat3(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0));
}
mat4 xlat_lib_saturate(mat4 m)
{
return mat4(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0), clamp(m[3], 0.0, 1.0));
}
vec4 xlat_main(in vec2 uv)
{
vec4 originalColor;
vec3 rgb;
originalColor = texture2D(sample0, uv);
rgb = (originalColor.xyz / originalColor.w);
rgb = xlat_lib_saturate(((rgb - threshold) / (1.00000 - threshold)));
return vec4((rgb * originalColor.w), originalColor.w);
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.7. brightness¶
- name
brightness.ps
- variable
contrast
- min
0
- max
2
- default
1.5
- variable
brightness
- min
0
- max
1
- default
0.5
- shader code
precision mediump float;
uniform float brightness;
uniform float contrast;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv,in vec4 pixelColor)
{
pixelColor.xyz /= pixelColor.w;
pixelColor.xyz = (((pixelColor.xyz - 0.500000) * max(contrast, 0.000000)) + 0.500000);
pixelColor.xyz += brightness;
pixelColor.xyz *= pixelColor.w;
return pixelColor;
}
void main()
{
vec4 color = texture2D(sample0, vTexCoord);
if (color.a == 0.0)
discard;
else gl_FragColor = xlat_main(vTexCoord,color);
}
13.2.8. color it¶
- name
color it.ps
- variable
color
- min
0, 0, 0
- max
1, 1, 1
- default
1, 0, 0
- variable
enable
- min
0
- max
1
- default
1
- shader code
precision mediump float;
uniform sampler2D sample0;
uniform vec3 color;
uniform float enable;
varying vec2 vTexCoord;
void main()
{
vec4 c = texture2D( sample0, vTexCoord.xy );
if(enable > 0.5)
gl_FragColor = vec4(color.r,color.g,color.b,c.a);
else
gl_FragColor = c;
}
13.2.9. color keying¶
- name
color keying.ps
- variable
colorDst
- min
0.1, 0.2, 0.3, 0
- max
1, 1, 1, 1
- default
1, 0.2, 1, 1
- variable
granThen
- min
0
- max
1
- default
1
- variable
colorSrc
- min
0.0392157, 0.0784314, 0.117647, 1
- max
0.490196, 0.501961, 0.980392, 1
- default
0.490196, 0.501961, 0.196078, 1
- variable
tolerance
- min
0
- max
1
- default
0.3
- shader code
precision mediump float;
uniform vec4 colorDst;
uniform vec4 colorSrc;
uniform float granThen;
uniform sampler2D sample0;
uniform float tolerance;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv, vec4 color)
{
if ((granThen > 0.500000))
{
if (all(lessThan(abs((color.xyz - colorSrc.xyz)), vec3(tolerance))))
{
color.xyzw = colorDst;
}
}
else
{
if (all(greaterThan(abs((color.xyz - colorSrc.xyz)), vec3(tolerance))))
{
color.xyzw = colorDst;
}
}
return color;
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
if ((color.w == 0.0))
discard;
else
gl_FragColor = xlat_main(vTexCoord, color);
}
13.2.10. color tone¶
- name
color tone.ps
- variable
desaturation
- min
0
- max
1
- default
0.5
- variable
lightColor
- min
0, 0, 0, 0
- max
1, 1, 1, 1
- default
1, 1, 1, 1
- variable
toned
- min
0
- max
1
- default
0.5
- variable
darkColor
- min
0, 0, 0, 0
- max
1, 1, 1, 1
- default
1, 1, 0, 0.7
- shader code
precision mediump float;
uniform vec4 darkColor;
uniform float desaturation;
uniform vec4 lightColor;
uniform sampler2D sample0;
uniform float toned;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv,in vec4 color)
{
vec3 scnColor;
float gray;
vec3 muted;
vec3 middle;
color = texture2D(sample0, uv);
scnColor = (vec3(lightColor) * (color.xyz / color.w));
gray = dot(vec3(0.300000, 0.590000, 0.110000), scnColor);
muted = mix(scnColor, vec3(vec3(gray)), vec3(desaturation));
middle = vec3(mix(darkColor, lightColor, vec4(gray)));
scnColor = mix(muted, middle, vec3(toned));
return vec4((scnColor * color.w), color.w);
}
void main()
{
vec4 color = texture2D(sample0, vTexCoord);
if (color.a == 0.0)
discard;
else gl_FragColor = xlat_main(vTexCoord,color);
}
13.2.11. edge gradient magnitude¶
- name
edge gradient magnitude.ps
- variable
imageSize
- min
0, 0
- max
1024, 1024
- default
256, 256
- variable
tolerance
- min
0
- max
1
- default
0
- shader code
precision mediump float;
uniform vec2 imageSize;
uniform sampler2D sample0;
uniform float tolerance;
varying vec2 vTexCoord;
vec4 xlat_main( in vec2 uv)
{
vec2 offsetTexture;
vec2 pixel_Right;
vec2 pixel_Left;
vec2 pixel_Top;
vec2 pixel_Bottom;
vec2 gradient;
float a;
offsetTexture = (1.00000 / imageSize);
pixel_Right = (uv.xy + vec2( offsetTexture.x , 0.000000));
pixel_Left = (uv.xy + vec2( ( -offsetTexture.x ), 0.000000));
pixel_Top = (uv.xy + vec2( 0.000000, offsetTexture.y ));
pixel_Bottom = (uv.xy + vec2( 0.000000, ( -offsetTexture.y )));
gradient = vec2( length( (texture2D( sample0, pixel_Right).xyz - texture2D( sample0, pixel_Left).xyz ) ), length( (texture2D( sample0, pixel_Top).xyz - texture2D( sample0, pixel_Bottom).xyz ) ));
a = length( gradient );
return vec4( a, a, a, 1.00000);
}
void main()
{
float a = texture2D(sample0, vTexCoord).a;
if (a == 0.0)
discard;
else
{
vec4 color = xlat_main(vTexCoord);
if(color.r <= tolerance && color.g <= tolerance && color.b <= tolerance)
discard;
else
gl_FragColor = xlat_main(vTexCoord);
}}
13.2.12. embossed¶
- name
embossed.ps
- variable
width
- min
0
- max
0.1
- default
0.0023
- variable
amount
- min
0
- max
1
- default
0.5
- shader code
precision mediump float;
uniform float amount;
uniform sampler2D sample0;
uniform float width;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv)
{
vec4 outC = vec4(0.500000, 0.500000, 0.500000, 1.00000);
outC -= (texture2D(sample0, (uv - width)) * amount);
outC += (texture2D(sample0, (uv + width)) * amount);
outC.xyz = vec3((((outC.x + outC.y) + outC.z) / 3.00000));
return outC;
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
if (color.a == 0.0)
discard;
else
gl_FragColor = xlat_main(vTexCoord);
}
13.2.13. explosion gaussian¶
- name
explosion gaussian.ps
- variable
sigma
- min
-2
- max
2
- default
0.15
- variable
color
- min
0, 0, 0
- max
1, 1, 1
- default
1, 1, 1
- variable
center
- min
-1, -1
- max
1.5, 1.5
- default
0.5, 0.5
- shader code
precision mediump float;
uniform sampler2D sample0;
uniform vec2 center;
uniform vec3 color;
uniform float sigma;
varying vec2 vTexCoord;
vec4 xlat_main( in vec2 uv )
{
float alpha;
float PI = 3.14152;
float s;
float q;
float a;
s = (2.0 * pow( sigma, 2.0));
q = (1.0 / (PI * s));
a = (q * exp( (( -(pow( (uv.x - center.x), 2.0) + pow( (uv.y - center.y), 2.0)) ) / s) ));
alpha = texture2D(sample0, uv.xy).a;
return vec4( a * color.r, a * color.g, a * color.b, alpha);
}
void main()
{
gl_FragColor = xlat_main( vTexCoord);
}
13.2.14. fade radial¶
- name
fade radial.ps
- variable
progress
- min
0
- max
100
- default
30
- shader code
precision mediump float;
uniform float progress;
uniform sampler2D sample0;
uniform sampler2D sample1;
varying vec2 vTexCoord;
vec4 RadialBlur(in vec4 color, in float progress, in vec2 uv)
{
vec2 center = vec2(0.500000, 0.500000);
vec2 toUV;
vec2 normToUV;
vec4 c1 = vec4(0.000000, 0.000000, 0.000000, 0.000000);
float s;
int i = 0;
toUV = (uv - center);
normToUV = toUV;
s = (progress * 0.0200000);
for (; (i < 24); (++i))
{
c1 += texture2D(sample1, (uv - ((normToUV * s) * float(i))));
}
c1 /= 24.0000;
return mix(c1, color, vec4(progress));
}
vec4 xlat_main(in vec2 uv)
{
vec4 color;
color = texture2D(sample0, uv);
if ((color.w == 0.000000))
{
return color;
}
return RadialBlur(color, (progress / 100.000), uv);
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.15. fade ripple¶
- name
fade ripple.ps
- variable
progress
- min
0
- max
100
- default
30
- shader code
precision mediump float;
uniform float progress;
uniform sampler2D sample0;
uniform sampler2D sample1;
varying vec2 vTexCoord;
vec4 Ripple(in float progress, in vec2 uv)
{
float frequency = 20.0000;
float speed = 10.0000;
float amplitude = 0.0500000;
vec2 center = vec2(0.500000, 0.500000);
vec2 toUV;
float distanceFromCenter;
vec2 normToUV;
float wave;
float offset1;
float offset2;
vec2 newUV1;
vec2 newUV2;
vec4 c1;
vec4 c2;
toUV = (uv - center);
distanceFromCenter = length(toUV);
normToUV = (toUV / distanceFromCenter);
wave = cos(((frequency * distanceFromCenter) - (speed * progress)));
offset1 = ((progress * wave) * amplitude);
offset2 = (((1.00000 - progress) * wave) * amplitude);
newUV1 = (center + (normToUV * (distanceFromCenter + offset1)));
newUV2 = (center + (normToUV * (distanceFromCenter + offset2)));
c1 = texture2D(sample1, newUV1);
c2 = texture2D(sample0, newUV2);
return mix(c1, c2, vec4(progress));
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
gl_FragColor = Ripple((progress / 100.000), vTexCoord);
}
13.2.16. fade saturate¶
- name
fade saturate.ps
- variable
progress
- min
0
- max
100
- default
30
- shader code
precision mediump float;
uniform float progress;
uniform sampler2D sample0;
uniform sampler2D sample1;
varying vec2 vTexCoord;
vec4 xlat_lib_saturate(vec4 x)
{
return clamp(x, 0.0, 1.0);
}
vec4 Saturate(in vec2 uv, in float progress, in vec4 c2)
{
vec4 c1;
float new_progress;
c1 = texture2D(sample1, uv);
c1 = xlat_lib_saturate((c1 * ((2.00000 * progress) + 1.00000)));
if ((progress > 0.800000))
{
new_progress = ((progress - 0.800000) * 5.00000);
return mix(c1, c2, vec4(new_progress));
}
else
{
return c1;
}
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
gl_FragColor = Saturate(vTexCoord, (progress / 100.000), color);
}
13.2.17. fade twist grid¶
- name
fade twist grid.ps
- variable
twistAmount
- min
-70
- max
70
- default
30
- variable
progress
- min
0
- max
100
- default
30
- shader code
precision mediump float;
uniform sampler2D sample0;
uniform sampler2D sample1;
uniform float progress;
uniform float twistAmount;
varying vec2 vTexCoord;
void xlat_lib_sincos(float x, out float s, out float c)
{
s = sin(x);
c = cos(x);
}
vec4 SwirlGrid(in vec2 uv, in float progress, in vec4 color)
{
float cellsize = 0.100000;
vec2 cell;
vec2 oddeven;
float cellTwistAmount;
vec2 newUV;
vec2 center = vec2(0.500000, 0.500000);
vec2 toUV;
float distanceFromCenter;
vec2 normToUV;
float angle;
vec2 newUV2;
vec4 c1;
cell = floor((uv * 10.0000));
oddeven = mod(cell, vec2(2.00000));
cellTwistAmount = twistAmount;
if ((oddeven.x < 1.00000))
{
cellTwistAmount *= -1.00000;
}
if ((oddeven.y < 1.00000))
{
cellTwistAmount *= -1.00000;
}
newUV = fract((uv * 10.0000));
toUV = (newUV - center);
distanceFromCenter = length(toUV);
normToUV = (toUV / distanceFromCenter);
angle = atan(normToUV.y, normToUV.x);
angle += (((distanceFromCenter * distanceFromCenter) * cellTwistAmount) * progress);
xlat_lib_sincos(angle, newUV2.y, newUV2.x);
newUV2 *= distanceFromCenter;
newUV2 += center;
newUV2 *= cellsize;
newUV2 += (cell * cellsize);
c1 = texture2D(sample1, newUV2);
return mix(c1, color, vec4(progress));
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
gl_FragColor = SwirlGrid(vTexCoord, (progress / 100.000), color);
}
13.2.18. fade twist¶
- name
fade twist.ps
- variable
twistAmount
- min
-70
- max
70
- default
30
- variable
progress
- min
0
- max
100
- default
30
- shader code
precision mediump float;
uniform float progress;
uniform sampler2D sample0;
uniform sampler2D sample1;
uniform float twistAmount;
varying vec2 vTexCoord;
void xlat_lib_sincos(float x, out float s, out float c)
{
s = sin(x);
c = cos(x);
}
vec2 xlat_lib_saturate(vec2 x)
{
return clamp(x, 0.0, 1.0);
}
vec4 SampleWithBorder(in vec4 border, in sampler2D tex, in vec2 uv)
{
if (any(bvec2((xlat_lib_saturate(uv) - uv))))
{
return border;
}
else
{
return texture2D(tex, uv);
}
}
vec4 Swirl(in vec2 uv, in float progress, in vec4 color)
{
vec2 center = vec2(0.500000, 0.500000);
vec2 toUV;
float distanceFromCenter;
vec2 normToUV;
float angle;
vec2 newUV;
vec4 c1;
toUV = (uv - center);
distanceFromCenter = length(toUV);
normToUV = (toUV / distanceFromCenter);
angle = atan(normToUV.y, normToUV.x);
angle += (((distanceFromCenter * distanceFromCenter) * twistAmount) * progress);
xlat_lib_sincos(angle, newUV.y, newUV.x);
newUV *= distanceFromCenter;
newUV += center;
c1 = SampleWithBorder(vec4(0.000000, 0.000000, 0.000000, 0.000000), sample1, newUV);
return mix(c1, color, vec4(progress));
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
gl_FragColor = Swirl(vTexCoord, (progress / 100.000), color);
}
13.2.19. fade wave¶
- name
fade wave.ps
- variable
progress
- min
0
- max
100
- default
30
- shader code
precision mediump float;
uniform float progress;
uniform sampler2D sample0;
uniform sampler2D sample1;
varying vec2 vTexCoord;
vec2 xlat_lib_saturate(vec2 x)
{
return clamp(x, 0.0, 1.0);
}
vec4 SampleWithBorder(in vec4 border, in sampler2D tex, in vec2 uv)
{
if (any(bvec2((xlat_lib_saturate(uv) - uv))))
{
return border;
}
else
{
return texture2D(tex, uv);
}
}
vec4 Wave(in vec2 uv, in float progress, in vec4 color)
{
float mag = 0.100000;
float phase = 14.0000;
float freq = 20.0000;
vec2 newUV;
vec4 c1;
newUV = (uv + vec2(((mag * progress) * sin(((freq * uv.y) + (phase * progress)))), 0.000000));
c1 = SampleWithBorder(vec4(0.000000), sample1, newUV);
return mix(c1, color, vec4(progress));
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
gl_FragColor = Wave(vTexCoord, (progress / 100.000), color);
}
13.2.20. fade¶
- name
fade.ps
- variable
progress
- min
0
- max
100
- default
30
- shader code
precision mediump float;
uniform float progress;
uniform sampler2D sample0;
uniform sampler2D sample1;
varying vec2 vTexCoord;
vec4 fade(vec4 c2)
{
vec4 c1 = texture2D(sample1, vTexCoord);
return mix(c1, c2, vec4(progress/100.0));
}
void main()
{
vec4 color0 = texture2D(sample0, vTexCoord);
gl_FragColor = fade(color0);
}
13.2.21. font¶
- name
font.ps
- variable
colorFont
- min
0, 0, 0
- max
1, 1, 1
- default
1, 1, 1
- shader code
precision mediump float;
uniform sampler2D sample0;
uniform vec3 colorFont;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv)
{
vec4 color;
color = texture2D(sample0, uv);
vec3 c2 = vec3(1.0 - colorFont.r,1.0 - colorFont.g,1.0 - colorFont.b);
color.rgb -= c2;
return color;
}
void main()
{
vec4 xlat_retVal;
xlat_retVal = xlat_main(vTexCoord);
gl_FragColor = vec4(xlat_retVal);
}
13.2.22. frosty out line¶
- name
frosty out line.ps
- variable
height
- min
0
- max
500
- default
300
- variable
width
- min
0
- max
650
- default
300
- shader code
precision mediump float;
uniform float height;
uniform sampler2D sample0;
uniform float width;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 middle)
{
vec2 topLeft;
vec2 top;
vec2 topRight;
vec2 left;
vec2 right;
vec2 bottomLeft;
vec2 bottom;
vec2 bottomRight;
vec4 m;
vec4 tl;
vec4 l;
vec4 bl;
vec4 t;
vec4 b;
vec4 tr;
vec4 r;
vec4 br;
vec4 color;
vec4 color2;
float avg;
topLeft.x = (middle.x - (1.00000 / width));
topLeft.y = (middle.y - (1.00000 / height));
top.x = middle.x;
top.y = (middle.y - (1.00000 / height));
topRight.x = (middle.x + (1.00000 / width));
topRight.y = (middle.y - (1.00000 / height));
left.x = (middle.x - (1.00000 / width));
left.y = middle.y;
right.x = (middle.x + (1.00000 / width));
right.y = middle.y;
bottomLeft.x = (middle.x - (1.00000 / width));
bottomLeft.y = (middle.y + (1.00000 / height));
bottom.x = middle.x;
bottom.y = (middle.y + (1.00000 / height));
bottomRight.x = (middle.x + (1.00000 / width));
bottomRight.y = (middle.y + (1.00000 / height));
m = texture2D(sample0, middle);
tl = texture2D(sample0, topLeft);
l = texture2D(sample0, left);
bl = texture2D(sample0, bottomLeft);
t = texture2D(sample0, top);
b = texture2D(sample0, bottom);
tr = texture2D(sample0, topRight);
r = texture2D(sample0, right);
br = texture2D(sample0, bottomRight);
color = (((((-tl) - t) - tr) + (((-l) + (8.00000 * m)) - r)) + (((-bl) - b) - br));
color2 = texture2D(sample0, middle);
avg = ((color.x + color.y) + color.z);
avg /= 3.00000;
color.xyz = vec3(avg);
color.w = 1.00000;
return (color2 + color);
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
if(color.a == 0.0)
discard;
else
gl_FragColor = xlat_main(vTexCoord);
}
13.2.23. glass tile¶
- name
glass tile.ps
- variable
bevelWidth
- min
1
- max
10
- default
10
- variable
offset
- min
0
- max
3
- default
3
- variable
groutColor
- min
0, 0, 0, 0
- max
1, 1, 1, 1
- default
0, 0, 0, 0
- variable
tiles
- min
0
- max
20
- default
5
- shader code
precision mediump float;
uniform float bevelWidth;
uniform vec4 groutColor;
uniform float offset;
uniform sampler2D sample0;
uniform float tiles;
varying vec2 vTexCoord;
void main()
{
float a = texture2D(sample0, vTexCoord).a;
if(a == 0.0 && groutColor.a == 0.0)
discard;
else
{
vec2 newUV1;
vec4 c1;
newUV1.xy = (vTexCoord.xy + (tan((((tiles * 2.50000) * vTexCoord.xy) + offset)) * (bevelWidth / 100.000)));
c1 = texture2D(sample0, newUV1);
if(c1.a == 0.0 && groutColor.a == 0.0)
discard;
else
{
if (((((newUV1.x < 0.000000) || (newUV1.x > 1.00000)) || (newUV1.y < 0.000000)) || (newUV1.y > 1.00000)))
{
c1 = groutColor;
}
if(c1.a == 0.0 && groutColor.a == 0.0)
discard;
else
{
gl_FragColor = c1;
}
}
}
}
13.2.24. invert color¶
- name
invert color.ps
- shader code
precision mediump float;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv)
{
vec4 color;
vec4 invertedColor;
color = texture2D(sample0, uv);
invertedColor = vec4((color.w - color.xyz), color.w);
return invertedColor;
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.25. luminance¶
- name
luminance.ps
- variable
color
- min
0, 0, 0, 0
- max
1, 1, 1, 1
- default
0.5, 0.5, 0.5, 0.5
- shader code
precision mediump float;
uniform vec4 color;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main( in vec2 uv )
{
vec4 texColor;
float luminance;
vec4 xlat_var_output;
vec4 white = vec4( 1.00000, 1.00000, 1.00000, 1.00000);
texColor = texture2D( sample0, uv);
luminance = dot( texColor, vec4( 0.212600, 0.715200, 0.0722000, 0.000000));
xlat_var_output = vec4( 0.000000);
if ( (luminance < 0.500000) ){
xlat_var_output = ((2.00000 * texColor) * color);
}
else{
xlat_var_output = (white - ((2.00000 * (white - texColor)) * (white - color)));
}
xlat_var_output.w = (texColor.w * color.w );
return xlat_var_output;
}
void main()
{
gl_FragColor = xlat_main( vTexCoord);
}
13.2.26. magnifying glass¶
- name
magnifying glass.ps
- variable
center
- min
0, 0
- max
1, 1
- default
0.5, 0.5
- variable
magnification
- min
0
- max
5
- default
2
- variable
aspectRatio
- min
0.5
- max
2
- default
0.94
- variable
radius
- min
0
- max
1
- default
0.25
- shader code
precision mediump float;
uniform float aspectRatio;
uniform vec2 center;
uniform float magnification;
uniform float radius;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv)
{
vec2 centerToPixel;
float dist;
vec2 samplePoint;
centerToPixel = (uv - center);
dist = length((centerToPixel / vec2(1.00000, aspectRatio)));
samplePoint = uv;
if ((dist < radius))
{
samplePoint = (center + (centerToPixel / magnification));
}
return texture2D(sample0, samplePoint);
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
if (color.a == 0.0)
discard;
else
gl_FragColor = xlat_main(vTexCoord);
}
13.2.27. multi textura¶
- name
multi textura.ps
- variable
gamma
- min
0
- max
100
- default
2
- shader code
precision mediump float;
varying vec2 vTexCoord;
uniform float gamma;
uniform sampler2D sample0;
uniform sampler2D sample1;
vec4 xlat_lib_saturate( vec4 x)
{
return clamp( x, 0.0, 1.0);
}
void main()
{
vec4 color1;
vec4 color2;
vec4 blendColor;
color1 = texture2D( sample0, vTexCoord.xy );
color2 = texture2D( sample1, vTexCoord.xy );
blendColor = ((color1 * color2) * gamma);
blendColor = xlat_lib_saturate( blendColor );
gl_FragColor = blendColor;
}
13.2.28. night vision blur¶
- name
night vision blur.ps
- variable
contrast
- min
1e-05
- max
10
- default
6.8
- variable
brightness
- min
1e-05
- max
100
- default
10
- shader code
precision mediump float;
uniform float brightness;
uniform float contrast;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec4 pixelColor)
{
vec4 color0;
color0 = pixelColor;
pixelColor = vec4((((0.299000 * pixelColor.x) + (0.587000 * pixelColor.y)) + (0.184000 * pixelColor.z)));
pixelColor.xyz /= pixelColor.w;
pixelColor.xyz = (((pixelColor.xyz - 0.500000) * max(contrast, 0.000000)) + 0.500000);
pixelColor.y += brightness;
pixelColor.xyz *= pixelColor.w;
return ((pixelColor * color0) * 0.250000);
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
if(color.a == 0.0)
discard;
else
gl_FragColor = xlat_main(color);
}
13.2.29. night vision¶
- name
night vision.ps
- variable
fInverseViewportHeight
- min
1e-05
- max
1
- default
1
- variable
fInverseViewportWidth
- min
1e-05
- max
1
- default
1
- shader code
precision mediump float;
uniform float fInverseViewportHeight;
uniform float fInverseViewportWidth;
uniform sampler2D sample0;
varying vec2 vTexCoord;
void main()
{
vec4 col;
vec4 color0;
col = texture2D(sample0, vTexCoord);
color0 = col;
col += (0.0625000 * texture2D(sample0, (vTexCoord + vec2((-1.00000 * fInverseViewportWidth), (-1.00000 * fInverseViewportHeight)))));
col += (0.0625000 * texture2D(sample0, (vTexCoord + vec2((-1.00000 * fInverseViewportWidth), (1.00000 * fInverseViewportHeight)))));
col += (0.0625000 * texture2D(sample0, (vTexCoord + vec2((1.00000 * fInverseViewportWidth), (-1.00000 * fInverseViewportHeight)))));
col += (0.0625000 * texture2D(sample0, (vTexCoord + vec2((1.00000 * fInverseViewportWidth), (1.00000 * fInverseViewportHeight)))));
col += (0.125000 * texture2D(sample0, (vTexCoord + vec2((-1.00000 * fInverseViewportWidth), (0.000000 * fInverseViewportHeight)))));
col += (0.125000 * texture2D(sample0, (vTexCoord + vec2((1.00000 * fInverseViewportWidth), (0.000000 * fInverseViewportHeight)))));
col += (0.125000 * texture2D(sample0, (vTexCoord + vec2((0.000000 * fInverseViewportWidth), (-1.00000 * fInverseViewportHeight)))));
col += (0.125000 * texture2D(sample0, (vTexCoord + vec2((0.000000 * fInverseViewportWidth), (1.00000 * fInverseViewportHeight)))));
col += (0.250000 * texture2D(sample0, (vTexCoord + vec2((0.000000 * fInverseViewportWidth), (0.000000 * fInverseViewportHeight)))));
col = vec4((((0.299000 * col.x) + (0.587000 * col.y)) + (0.184000 * col.z)));
col = vec4(col.xxx, col.w);
col.y *= 3.00000;
col = ((col * color0) * 0.500000);
gl_FragColor = col;
}
13.2.30. old movie¶
- name
old movie.ps
- variable
noiseAmount
- min
1e-05
- max
1
- default
0.0001
- variable
scratchAmount
- min
1e-05
- max
0.1
- default
0.044
- variable
frame
- min
0
- max
2
- default
1
- shader code
precision mediump float;
uniform float frame;
uniform float noiseAmount;
uniform sampler2D sample0;
uniform float scratchAmount;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv,in vec4 color)
{
float ScratchAmountInv;
vec2 sc;
float scratch;
vec2 rCoord;
vec3 rand;
float gray;
vec2 dist;
ScratchAmountInv = (1.00000 / scratchAmount);
sc = (frame * vec2(0.00100000, 0.400000));
sc.x = fract((uv.x + sc.x));
scratch = sc.x;
scratch = ((2.00000 * scratch) * ScratchAmountInv);
scratch = (1.00000 - abs((1.00000 - scratch)));
scratch = max(0.000000, scratch);
color.xyz += vec3(scratch);
rCoord = (uv * 0.330000);
rand = vec3(texture2D(sample0, rCoord));
if ((noiseAmount > rand.x))
{
color.xyz = vec3((0.100000 + (rand.z * 0.400000)));
}
gray = dot(color, vec4(0.300000, 0.590000, 0.110000, 0.000000));
color = vec4((gray * vec3(0.900000, 0.800000, 0.600000)), 1.00000);
dist = (0.500000 - uv);
color.xyz *= ((0.400000 - dot(dist, dist)) * 2.80000);
return color;
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
if(color.a == 0.0)
discard;
else
gl_FragColor = xlat_main(vTexCoord,color);
}
13.2.31. out of bounds¶
- name
out of bounds.ps
- variable
color
- min
0, 0, 0
- max
1, 1, 1
- default
1, 0, 0
- shader code
precision mediump float;
uniform sampler2D sample0;
uniform vec3 color;
varying vec2 vTexCoord;
void main()
{
vec4 colorRet = texture2D( sample0, vTexCoord.xy );
if(vTexCoord.x < 0.0 || vTexCoord.x > 1.0 || vTexCoord.y < 0.0 || vTexCoord.y > 1.0)
colorRet.rgb *= color.rgb;
gl_FragColor = colorRet;
}
13.2.32. pinch mouse¶
- name
pinch mouse.ps
- variable
strength
- min
0
- max
2
- default
1
- variable
center
- min
0, 0
- max
794, 678
- default
0.5, 0.5
- variable
aspectRatio
- min
0.5
- max
2
- default
1
- variable
radius
- min
0
- max
1
- default
0.25
- shader code
precision mediump float;
uniform float aspectRatio;
uniform vec2 center;
uniform float radius;
uniform sampler2D sample0;
uniform float strength;
varying vec2 vTexCoord;
float xlat_lib_saturate(float x)
{
return clamp(x, 0.0, 1.0);
}
vec2 xlat_lib_saturate(vec2 x)
{
return clamp(x, 0.0, 1.0);
}
vec3 xlat_lib_saturate(vec3 x)
{
return clamp(x, 0.0, 1.0);
}
vec4 xlat_lib_saturate(vec4 x)
{
return clamp(x, 0.0, 1.0);
}
mat2 xlat_lib_saturate(mat2 m)
{
return mat2(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0));
}
mat3 xlat_lib_saturate(mat3 m)
{
return mat3(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0));
}
mat4 xlat_lib_saturate(mat4 m)
{
return mat4(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0), clamp(m[3], 0.0, 1.0));
}
vec4 xlat_main(in vec2 uv)
{
vec2 newCenter;
vec2 dir;
vec2 scaledDir;
float dist;
float range;
vec2 samplePoint;
newCenter.x = (1.00000 - (center.x / 794.000));
newCenter.y = (1.00000 - (center.y / 678.000));
dir = (newCenter - uv);
scaledDir = dir;
scaledDir.y /= aspectRatio;
dist = length(scaledDir);
range = xlat_lib_saturate((1.00000 - (dist / (abs(((-sin((radius * 8.00000))) * radius)) + 1.00000e-008))));
samplePoint = (uv + ((dir * range) * strength));
return texture2D(sample0, samplePoint);
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.33. pinch¶
- name
pinch.ps
- variable
strength
- min
0
- max
2
- default
1
- variable
center
- min
0, 0
- max
1, 1
- default
0.5, 0.5
- variable
aspectRatio
- min
0.5
- max
2
- default
1
- variable
radius
- min
0
- max
1
- default
0.25
- shader code
precision mediump float;
uniform float aspectRatio;
uniform vec2 center;
uniform float radius;
uniform sampler2D sample0;
uniform float strength;
varying vec2 vTexCoord;
float xlat_lib_saturate(float x)
{
return clamp(x, 0.0, 1.0);
}
vec2 xlat_lib_saturate(vec2 x)
{
return clamp(x, 0.0, 1.0);
}
vec3 xlat_lib_saturate(vec3 x)
{
return clamp(x, 0.0, 1.0);
}
vec4 xlat_lib_saturate(vec4 x)
{
return clamp(x, 0.0, 1.0);
}
mat2 xlat_lib_saturate(mat2 m)
{
return mat2(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0));
}
mat3 xlat_lib_saturate(mat3 m)
{
return mat3(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0));
}
mat4 xlat_lib_saturate(mat4 m)
{
return mat4(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0), clamp(m[3], 0.0, 1.0));
}
vec4 xlat_main(in vec2 uv)
{
vec2 dir;
vec2 scaledDir;
float dist;
float range;
vec2 samplePoint;
dir = (center - uv);
scaledDir = dir;
scaledDir.y /= aspectRatio;
dist = length(scaledDir);
range = xlat_lib_saturate((1.00000 - (dist / (abs(((-sin((radius * 8.00000))) * radius)) + 1.00000e-008))));
samplePoint = (uv + ((dir * range) * strength));
return texture2D(sample0, samplePoint);
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.34. poisson¶
- name
poisson.ps
- variable
poisson
- min
1
- max
10
- default
3
- variable
inputSize
- min
1, 1
- max
300, 300
- default
100, 100
- shader code
precision mediump float;
uniform vec2 inputSize;
uniform float poisson;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv)
{
vec4 cOut;
vec2 coord;
cOut = texture2D(sample0, uv);
coord = (uv.xy + ((vec2(-0.326212, -0.405810) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(-0.840144, -0.07358) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(-0.695914, 0.457137) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(-0.203345, 0.620716) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(0.96234, -0.194983) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(0.473434, -0.480026) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(0.519456, 0.767022) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(0.185461, -0.893124) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(0.507431, 0.064425) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(0.89642, 0.412458) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(-0.32194, -0.932615) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
coord = (uv.xy + ((vec2(-0.791559, -0.59771) / inputSize) * poisson));
cOut += texture2D(sample0, coord);
return (cOut / 13.0000);
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.35. ripple¶
- name
ripple.ps
- variable
center
- min
0, 0
- max
1, 1
- default
0.5, 0.5
- variable
aspectRatio
- min
0.5
- max
2
- default
1.34
- variable
phase
- min
-20
- max
20
- default
0
- variable
frequency
- min
0
- max
100
- default
70
- variable
amplitude
- min
0
- max
1
- default
0.1
- shader code
precision mediump float;
uniform float amplitude;
uniform float aspectRatio;
uniform vec2 center;
uniform float frequency;
uniform float phase;
uniform sampler2D sample0;
varying vec2 vTexCoord;
void xlat_lib_sincos(float x, out float s, out float c)
{
s = sin(x);
c = cos(x);
}
void xlat_lib_sincos(vec2 x, out vec2 s, out vec2 c)
{
s = sin(x);
c = cos(x);
}
void xlat_lib_sincos(vec3 x, out vec3 s, out vec3 c)
{
s = sin(x);
c = cos(x);
}
void xlat_lib_sincos(vec4 x, out vec4 s, out vec4 c)
{
s = sin(x);
c = cos(x);
}
void xlat_lib_sincos(mat2 x, out mat2 s, out mat2 c)
{
s = mat2(sin(x[0]), sin(x[1]));
c = mat2(cos(x[0]), cos(x[1]));
}
void xlat_lib_sincos(mat3 x, out mat3 s, out mat3 c)
{
s = mat3(sin(x[0]), sin(x[1]), sin(x[2]));
c = mat3(cos(x[0]), cos(x[1]), cos(x[2]));
}
void xlat_lib_sincos(mat4 x, out mat4 s, out mat4 c)
{
s = mat4(sin(x[0]), sin(x[1]), sin(x[2]), sin(x[3]));
c = mat4(cos(x[0]), cos(x[1]), cos(x[2]), cos(x[3]));
}
float xlat_lib_saturate(float x)
{
return clamp(x, 0.0, 1.0);
}
vec2 xlat_lib_saturate(vec2 x)
{
return clamp(x, 0.0, 1.0);
}
vec3 xlat_lib_saturate(vec3 x)
{
return clamp(x, 0.0, 1.0);
}
vec4 xlat_lib_saturate(vec4 x)
{
return clamp(x, 0.0, 1.0);
}
mat2 xlat_lib_saturate(mat2 m)
{
return mat2(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0));
}
mat3 xlat_lib_saturate(mat3 m)
{
return mat3(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0));
}
mat4 xlat_lib_saturate(mat4 m)
{
return mat4(clamp(m[0], 0.0, 1.0), clamp(m[1], 0.0, 1.0), clamp(m[2], 0.0, 1.0), clamp(m[3], 0.0, 1.0));
}
vec4 xlat_main(in vec2 uv)
{
vec2 dir;
float dist;
vec2 wave;
float falloff;
vec2 samplePoint;
vec4 color;
float lighting;
dir = (uv - center);
dir.y /= aspectRatio;
dist = length(dir);
dir /= dist;
dir.y *= aspectRatio;
xlat_lib_sincos(((frequency * dist) + phase), wave.x, wave.y);
falloff = xlat_lib_saturate((1.00000 - dist));
falloff *= falloff;
dist += ((amplitude * wave.x) * falloff);
samplePoint = (center + (dist * dir));
color = texture2D(sample0, samplePoint);
lighting = (1.00000 - ((amplitude * 0.200000) * (1.00000 - xlat_lib_saturate((wave.y * falloff)))));
color.xyz *= lighting;
return color;
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.36. saturate¶
- name
saturate.ps
- variable
color
- min
0, 0, 0
- max
1, 1, 1
- default
1, 1, 1
- shader code
precision mediump float;
uniform vec3 color;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main( in vec2 uv )
{
vec4 c1;
c1 = texture2D( sample0, uv.xy );
c1.xyz *= color.xyz ;
return c1;
}
void main()
{
gl_FragColor = xlat_main( vTexCoord);
}
13.2.37. sharpen¶
- name
sharpen.ps
- variable
inputSize
- min
1, 1
- max
1000, 1000
- default
800, 600
- variable
amount
- min
0
- max
2
- default
1
- shader code
precision mediump float;
uniform float amount;
uniform vec2 inputSize;
uniform sampler2D sample0;
varying vec2 vTexCoord;
void main()
{
vec2 offset;
vec4 color;
offset = (1.00000 / inputSize);
color = texture2D(sample0, vTexCoord);
color.xyz += vec3((texture2D(sample0, (vTexCoord - offset)) * amount));
color.xyz -= vec3((texture2D(sample0, (vTexCoord + offset)) * amount));
gl_FragColor = color;
}
13.2.38. sketch¶
- name
sketch.ps
- variable
brushSize
- min
0.0006
- max
1
- default
0.003
- shader code
precision mediump float;
uniform sampler2D Image;
uniform float brushSize;
varying vec2 vTexCoord;
const vec2 sample1 = vec2(0.000000, -1.00000);
const vec2 sample2 = vec2(-1.00000, 0.000000);
const vec2 sample3 = vec2(1.00000, 0.000000);
const vec2 sample4 = vec2(0.000000, 1.00000);
vec4 xlat_main(in vec2 texCoord)
{
vec4 color;
vec4 laplace;
vec4 complement;
float gray;
float gray_1;
color = texture2D(Image, texCoord);
laplace = (-4.00000 * color);
laplace += texture2D(Image, (texCoord + (brushSize * sample1)));
laplace.x = float(laplace.xyz);
laplace.y = float(laplace.xyz);
laplace.z = float(laplace.xyz);
laplace += texture2D(Image, (texCoord + (brushSize * sample2)));
laplace.x = float(laplace.xyz);
laplace.y = float(laplace.xyz);
laplace.z = float(laplace.xyz);
laplace += texture2D(Image, (texCoord + (brushSize * sample3)));
laplace.x = float(laplace.xyz);
laplace.y = float(laplace.xyz);
laplace.z = float(laplace.xyz);
laplace += texture2D(Image, (texCoord + (brushSize * sample2)));
laplace.x = float(laplace.xyz);
laplace.y = float(laplace.xyz);
laplace.z = float(laplace.xyz);
laplace = (1.00000 / laplace);
complement.xyz = (1.00000 - laplace.xyz);
complement.w = color.w;
if ((complement.x > 1.00000))
{
gray = (((complement.x * 0.300000) + (complement.y * 0.590000)) + (complement.z * 0.110000));
complement.x = gray;
complement.y = gray;
complement.z = gray;
return complement;
}
else
{
gray_1 = (((color.x * 0.300000) + (color.y * 0.590000)) + (color.z * 0.110000));
color.x = gray_1;
color.y = gray_1;
color.z = gray_1;
return color;
}
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.39. smooth magnify¶
- name
smooth magnify.ps
- variable
center
- min
0, 0
- max
1, 1
- default
0.5, 0.5
- variable
aspectRatio
- min
0.5
- max
2
- default
1.4
- variable
innerRadius
- min
0
- max
1
- default
0.2
- variable
magnification
- min
0
- max
5
- default
2
- variable
outerRadius
- min
0
- max
1
- default
0.4
- shader code
precision mediump float;
uniform float aspectRatio;
uniform vec2 center;
uniform float innerRadius;
uniform float magnification;
uniform float outerRadius;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv)
{
vec2 centerToPixel;
float dist;
float ratio;
vec2 samplePoint;
centerToPixel = (uv - center);
dist = length((centerToPixel / vec2(1.00000, aspectRatio)));
ratio = smoothstep(innerRadius, max(innerRadius, outerRadius), dist);
samplePoint = mix((center + (centerToPixel / magnification)), uv, vec2(ratio));
return texture2D(sample0, samplePoint);
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.40. spiral¶
- name
spiral.ps
- variable
center
- min
0, 0
- max
1, 1
- default
0.5, 0.5
- variable
aspectRatio
- min
0.5
- max
2
- default
1.4
- variable
spiralStrength
- min
0
- max
20
- default
10
- shader code
precision mediump float;
uniform float aspectRatio;
uniform vec2 center;
uniform sampler2D sample0;
uniform float spiralStrength;
varying vec2 vTexCoord;
void xlat_lib_sincos(float x, out float s, out float c)
{
s = sin(x);
c = cos(x);
}
void xlat_lib_sincos(vec2 x, out vec2 s, out vec2 c)
{
s = sin(x);
c = cos(x);
}
void xlat_lib_sincos(vec3 x, out vec3 s, out vec3 c)
{
s = sin(x);
c = cos(x);
}
void xlat_lib_sincos(vec4 x, out vec4 s, out vec4 c)
{
s = sin(x);
c = cos(x);
}
void xlat_lib_sincos(mat2 x, out mat2 s, out mat2 c)
{
s = mat2(sin(x[0]), sin(x[1]));
c = mat2(cos(x[0]), cos(x[1]));
}
void xlat_lib_sincos(mat3 x, out mat3 s, out mat3 c)
{
s = mat3(sin(x[0]), sin(x[1]), sin(x[2]));
c = mat3(cos(x[0]), cos(x[1]), cos(x[2]));
}
void xlat_lib_sincos(mat4 x, out mat4 s, out mat4 c)
{
s = mat4(sin(x[0]), sin(x[1]), sin(x[2]), sin(x[3]));
c = mat4(cos(x[0]), cos(x[1]), cos(x[2]), cos(x[3]));
}
vec4 xlat_main(in vec2 uv)
{
vec2 dir;
float dist;
float angle;
float newAngle;
vec2 newDir;
vec2 samplePoint;
bool isValid;
dir = (uv - center);
dir.y /= aspectRatio;
dist = length(dir);
angle = atan(dir.y, dir.x);
newAngle = (angle + (spiralStrength * dist));
xlat_lib_sincos(newAngle, newDir.y, newDir.x);
newDir.y *= aspectRatio;
samplePoint = (center + (newDir * dist));
if ((all(greaterThanEqual(samplePoint, vec2(0.000000))) && all(lessThanEqual(samplePoint, vec2(1.00000)))))
{
isValid = true;
}
else
{
isValid = false;
}
return (isValid) ? (texture2D(sample0, samplePoint)) : (vec4(0.000000, 0.000000, 0.000000, 0.000000));
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.2.41. texture map¶
- name
texture map.ps
- variable
horizontalSize
- min
0
- max
5
- default
1
- variable
verticalOffset
- min
0
- max
1
- default
0
- variable
horizontalOffset
- min
0
- max
1
- default
0
- variable
strength
- min
0
- max
1
- default
0.3
- variable
verticalSize
- min
0
- max
5
- default
1
- shader code
precision mediump float;
uniform float horizontalOffset;
uniform float horizontalSize;
uniform sampler2D sample0;
uniform sampler2D sample1;
uniform float strength;
uniform float verticalOffset;
uniform float verticalSize;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv)
{
float horzOffset;
float vOffset;
vec2 offset;
vec4 c1;
horzOffset = fract(((uv.x / horizontalSize) + min(1.00000, horizontalOffset)));
vOffset = fract(((uv.y / verticalSize) + min(1.00000, verticalOffset)));
offset = ((texture2D(sample1, vec2(horzOffset, vOffset)).xy * strength) - (strength / 8.00000));
c1 = texture2D(sample0, fract((uv + offset)));
return c1;
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
gl_FragColor = xlat_main(vTexCoord);
}
13.2.42. tiled map¶
- name
tiled map.ps
- variable
alpha
- min
0
- max
1
- default
0
- variable
tolerance
- min
0
- max
1
- default
0.3
- variable
granThen
- min
0
- max
1
- default
1
- variable
colorSrc
- min
0, 0, 0
- max
1, 1, 1
- default
1, 0, 1
- variable
enableColorKeying
- min
0
- max
1
- default
0
- shader code
precision mediump float;
uniform float enableColorKeying;
uniform float granThen;
uniform float alpha;
uniform float tolerance;
uniform vec3 colorSrc;
uniform sampler2D sample0;
varying vec2 vTexCoord;
void main()
{
vec4 color = texture2D(sample0, vTexCoord);
color.a -= alpha;
if (color.a <= 0.0)
{
discard;
}
else if (enableColorKeying > 0.5)
{
if (granThen > 0.5)
{
if (all(lessThan(abs((color.xyz - colorSrc.xyz)), vec3(tolerance))))
{
discard;
}
else
{
gl_FragColor = color;
}
}
else
{
if (all(greaterThan(abs((color.xyz - colorSrc.xyz)), vec3(tolerance))))
{
discard;
}
else
{
gl_FragColor = color;
}
}
}
else
{
gl_FragColor = color;
}
}
13.2.43. tone mapping¶
- name
tone mapping.ps
- variable
fogColor
- min
0, 0, 0, 0
- max
1, 1, 1, 1
- default
1, 1, 1, 1
- variable
gamma
- min
0.5
- max
2
- default
0.63
- variable
vignetteCenter
- min
0, 0
- max
1, 1
- default
0.5, 0.5
- variable
blueShift
- min
0
- max
10
- default
10
- variable
vignetteAmount
- min
-1
- max
1
- default
0
- variable
defog
- min
0
- max
1
- default
0.4
- variable
exposure
- min
-1
- max
1
- default
-0.2
- variable
vignetteRadius
- min
0
- max
1
- default
0.5
- shader code
precision mediump float;
uniform float blueShift;
uniform float defog;
uniform float exposure;
uniform vec4 fogColor;
uniform float gamma;
uniform sampler2D sample0;
uniform float vignetteAmount;
uniform vec2 vignetteCenter;
uniform float vignetteRadius;
varying vec2 vTexCoord;
vec4 xlat_main(in vec2 uv, in vec4 c)
{
vec2 tc;
float v;
vec3 d;
c.xyz = vec3(max(0.000000, float((c.xyz - (defog * fogColor.xyz)))));
c.xyz *= pow(2.00000, exposure);
c.xyz = pow(c.xyz, vec3(gamma));
tc = (uv - vignetteCenter);
v = (length(tc) / vignetteRadius);
c.xyz += (pow(v, 4.00000) * vignetteAmount);
d = (c.xyz * vec3(1.05000, 0.970000, 1.27000));
c.xyz = mix(c.xyz, d, vec3(blueShift));
return c;
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
gl_FragColor = xlat_main(vTexCoord,color);
}
13.2.44. toon¶
- name
toon.ps
- variable
levels
- min
0
- max
15
- default
5
- shader code
precision mediump float;
uniform float levels;
uniform sampler2D sample0;
varying vec2 vTexCoord;
vec4 xlat_main(in vec4 color)
{
int result;
color.xyz /= color.w;
result = int(floor(levels));
color.xyz *= float(result);
color.xyz = floor(color.xyz);
color.xyz /= float(result);
color.xyz *= color.w;
return color;
}
void main()
{
vec4 color;
color = texture2D(sample0, vTexCoord);
if(color.a == 0.0)
discard;
else
gl_FragColor = xlat_main(color);
}
13.2.45. transparent¶
- name
transparent.ps
- variable
alpha
- min
0
- max
1
- default
0.7
- shader code
precision mediump float;
uniform float alpha;
uniform sampler2D sample0;
varying vec2 vTexCoord;
void main()
{
vec4 color;
color = texture2D( sample0, vTexCoord);
color.a -= alpha;
gl_FragColor = color;
}
13.2.46. wave¶
- name
wave.ps
- variable
effectTime
- min
0
- max
10
- default
0
- variable
sizeWave
- min
-50
- max
100
- default
10
- shader code
precision mediump float;
uniform sampler2D sample0;
uniform float effectTime;
uniform float sizeWave;
varying vec2 vTexCoord;
float dist(in float a, in float b, in float c, in float d)
{
return sqrt((((a - c) * (a - c)) + ((b - d) * (b - d))));
}
vec4 xlat_main(in vec2 uv)
{
vec4 Color;
float f;
Color = vec4(0.000000);
f = ((sin((dist((uv.x + effectTime), uv.y, 0.128000, 0.128000) * sizeWave)) + sin((dist(uv.x, uv.y, 0.640000, 0.640000) * sizeWave))) + sin((dist(uv.x, (uv.y + (effectTime / 7.00000)), 0.192000, 0.640000) * sizeWave)));
uv.xy = (uv.xy + (f / sizeWave));
Color = texture2D(sample0, uv.xy);
return Color;
}
void main()
{
gl_FragColor = xlat_main(vTexCoord);
}
13.3. vertex shader¶
Bellow all embedded vertex shader available for the engine:
13.3.1. scale¶
- name
scale.vs
- variable
scale
- min
0, 0, 0
- max
10, 10, 10
- default
1, 1, 1
- shader code
precision highp float;
uniform mat4 mvpMatrix;
uniform vec3 scale;
attribute vec4 aPosition;
attribute vec4 aNormal;
attribute vec2 aTextCoord;
varying vec2 vTexCoord;
struct VS_OUTPUT
{
vec4 pos;
vec2 uv;
};
VS_OUTPUT xlat_main(in vec4 pos, in vec4 nor, in vec2 uv)
{
VS_OUTPUT ret;
pos.x *= scale.x;
pos.y *= scale.y;
pos.z *= scale.z;
ret.pos = (mvpMatrix * pos);
ret.uv = uv;
return ret;
}
void main()
{
VS_OUTPUT ret;
ret = xlat_main(aPosition, aNormal, aTextCoord);
vTexCoord = ret.uv;
gl_Position = vec4(ret.pos);
}
13.3.2. simple texture¶
- name
simple texture.vs
- shader code
precision highp float;
uniform mat4 mvpMatrix;
attribute vec4 aPosition;
attribute vec4 aNormal;
attribute vec2 aTextCoord;
varying vec4 vPosition;
varying vec4 vNormal;
varying vec2 vTexCoord;
void main()
{
vPosition = aPosition;
vNormal = aNormal;
vTexCoord = aTextCoord;
gl_Position = (mvpMatrix * aPosition);
}