Table of Contents
10. Table particle¶
A table particle inherit from renderizable and implement all methods as usual.
A particle has a ‘stage’ which represent the animation. Each stage might have its own behavior. Also each stage has a shader table by default which can be accessed by getShader method.
Particles have a peculiarity state of being born
, living
and dying
and then resurrecting (or not).
This is well known in all generator of particles and on this engine could not be different.
There are several combinations that provide a set of options for reach this.
10.1. particle methods¶
10.1.1. particle new¶
- new(string * world, number * x, number * y, number * z)¶
Create a new instance of a particle passing the world desired (detail) and position.
- Parameters
string – world can be
2ds
,2dw
or3d
.number – x position (optional).
number – y position (optional).
number – z position (optional).
- Returns
particle table.
Example:
tParticle = particle:new('2dw') --note that particle inherits from renderizable
10.1.2. particle load¶
- load(string file_name, number * total_particle, string * shader_operator, string * aditional_code_shader)¶
Load a particle from file (expected
.plt
extension) any of images supported types.- Parameters
string – file name can be a texture directly or an binary file
.ptl
number – total_particle initial (optional).
string – shader operator can be
+
,-
,/
,*
, (optional).string – additional code initial (optional).
- Returns
boolean
- result.
Example:
tParticle = particle:new('2d')
if tParticle:load('particle.ptl',50,'*') then
print('Successfully loaded particle:','particle.ptl')
else
print('Failed to load particle:','particle.ptl')
end
Note
10.1.3. particle add¶
- add(number total_particle, boolean * force_now)¶
Add more particle to a particle system.
- Parameters
number – total_particle to be added.
boolean – force now means that all particle will appear instantaneously (optional).
- Returns
boolean
- result.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:add(1000) --add 1000 particles
else
print('Failed to load particle:','particle.ptl')
end
10.1.4. particle Offset¶
- setMinOffset(number stage, number * x, number * y, number * z)¶
Change the min offset from a particle system.
- Parameters
number – stage to be modified.
number – x value (optional).
number – y value (optional).
number – z value (optional).
Example:
tParticle = particle:new('2dw')
local stage = 1 --we only have one stage for this particle
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMinOffset(stage,-55,-150,0)
else
print('Failed to load particle:','particle.ptl')
end
- setMinOffset(number stage, vec3 minOffset)¶
Change the min offset from a particle system.
- Parameters
number – stage to be modified.
number – minOffset value as vec3.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local minOffset = vec3:new(-45,-100,0)
tParticle:setMinOffset(stage,minOffset)
else
print('Failed to load particle:','particle.ptl')
end
- getMinOffset(number stage)¶
Get the min offset from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
,number
,number
-x
,y
andz
min offset.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local x,y,z = tParticle:getMinOffset(stage)
print('Current min offset:',x,y,z)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxOffset(number stage, number * x, number * y, number * z)¶
Change the max offset from a particle system.
- Parameters
number – stage to be modified.
number – x value (optional).
number – y value (optional).
number – z value (optional).
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMaxOffset(stage,-55,-150,0)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxOffset(number stage, vec3 maxOffset)¶
Change the max offset from a particle system.
- Parameters
number – stage to be modified.
number – maxOffset value as vec3.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local maxOffset = vec3:new(-35,-120,0)
tParticle:setMaxOffset(stage,maxOffset)
else
print('Failed to load particle:','particle.ptl')
end
- getMaxOffset(number stage)¶
Get the max offset from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
,number
,number
-x
,y
andz
min offset.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local x,y,z = tParticle:getMaxOffset(stage)
print('Current max offset:',x,y,z)
else
print('Failed to load particle:','particle.ptl')
end
10.1.5. particle Direction¶
- setMinDirection(number stage, number * x, number * y, number * z)¶
Change the min direction from a particle system.
- Parameters
number – stage to be modified.
number – x value (optional).
number – y value (optional).
number – z value (optional).
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMinDirection(stage,-55,-150,0)
else
print('Failed to load particle:','particle.ptl')
end
- setMinDirection(number stage, vec3 minDirection)¶
Change the min direction from a particle system.
- Parameters
number – stage to be modified.
number – minDirection value as vec3.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local minDirection = vec3:new(-54,-154,0)
tParticle:setMinDirection(stage,minDirection)
else
print('Failed to load particle:','particle.ptl')
end
- getMinDirection(number stage)¶
Get the min direction from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
,number
,number
-x
,y
andz
min direction.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local x,y,z = tParticle:getMinDirection(stage)
print('Current min direction:',x,y,z)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxDirection(number stage, number * x, number * y, number * z)¶
Change the max direction from a particle system.
- Parameters
number – stage to be modified.
number – x value (optional).
number – y value (optional).
number – z value (optional).
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMaxDirection(stage,-55,-150,0)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxDirection(number stage, vec3 maxDirection)¶
Change the max direction from a particle system.
- Parameters
number – stage to be modified.
number – vec3 value as vec3.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local maxDirection = vec3:new(-55,-150,0)
tParticle:setMaxDirection(stage,maxDirection)
else
print('Failed to load particle:','particle.ptl')
end
- getMaxDirection(number stage)¶
Get the max direction from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
,number
,number
-x
,y
andz
min direction.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local x,y,z = tParticle:getMaxDirection(stage)
print('Current max direction:',x,y,z)
else
print('Failed to load particle:','particle.ptl')
end
10.1.6. particle Speed¶
- setMinSpeed(number stage, number min_speed)¶
Change the min speed from a particle system.
- Parameters
number – stage to be modified.
number – min_speed value.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMinSpeed(stage,500)
else
print('Failed to load particle:','particle.ptl')
end
- getMinSpeed(number stage)¶
Get the min speed from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
- min speed.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local min_speed = tParticle:getMinSpeed(stage)
print('Current min speed:',min_speed)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxSpeed(number stage, number max_speed)¶
Change the max speed from a particle system.
- Parameters
number – stage to be modified.
number – max_speed value.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMaxSpeed(stage,150)
else
print('Failed to load particle:','particle.ptl')
end
- getMaxSpeed(number stage)¶
Get the max speed from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
- max speed.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local max_speed = tParticle:getMaxSpeed(stage)
print('Current max speed:',max_speed)
else
print('Failed to load particle:','particle.ptl')
end
10.1.7. particle Color¶
- setMinColor(number stage, number * r, number * g, number * b)¶
Change the min color from a particle system.
- Parameters
number – stage to be modified.
number – r value
number – g value
number – b value
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMinColor(stage,0,0.5,0)
else
print('Failed to load particle:','particle.ptl')
end
- setMinColor(number stage, vec3 minColor)¶
Change the min color from a particle system.
- Parameters
number – stage to be modified.
number – minColor value as vec3.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local minColor = vec3:new(1,1,0.8)
tParticle:setMinColor(stage,minColor)
else
print('Failed to load particle:','particle.ptl')
end
- getMinColor(number stage)¶
Get the min color from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
,number
,number
-r
,g
andb
min color.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local r,g,b = tParticle:getMinColor(stage)
print('Current min color:',r,g,b)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxColor(number stage, number * r, number * g, number * b)¶
Change the max color from a particle system.
- Parameters
number – stage to be modified.
number – r value
number – g value
number – b value
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMaxColor(stage,1,1,1)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxColor(number stage, vec3 maxColor)¶
Change the max color from a particle system.
- Parameters
number – stage to be modified.
number – maxColor value as vec3.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local maxColor = vec3:new(1,1,1)
tParticle:setMaxColor(stage,maxColor)
else
print('Failed to load particle:','particle.ptl')
end
- getMaxColor(number stage)¶
Get the max color from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
,number
,number
-r
,g
andb
min color.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local r,g,b = tParticle:getMaxColor(stage)
print('Current max color:',r,g,b)
else
print('Failed to load particle:','particle.ptl')
end
10.1.8. particle LifeTime¶
- setMinLifeTime(number stage, number * time)¶
Change the min time from a particle system.
- Parameters
number – stage to be modified.
number – min time of life in seconds for each new particle.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMinLifeTime(stage,6.5)
else
print('Failed to load particle:','particle.ptl')
end
- getMinLifeTime(number stage)¶
Get the min time from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
-min
time of life in seconds.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local minTime = tParticle:getMinLifeTime(stage)
print('Current min time:',minTime)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxLifeTime(number stage, number * time)¶
Change the max time from a particle system.
- Parameters
number – stage to be modified.
number – max time of life in seconds.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMaxLifeTime(stage,5.5)
else
print('Failed to load particle:','particle.ptl')
end
- getMaxLifeTime(number stage)¶
Get the max life time from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
, max time of life in seconds.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local maxLifeTime = tParticle:getMaxLifeTime(stage)
print('Current max time:',maxLifeTime)
else
print('Failed to load particle:','particle.ptl')
end
10.1.9. particle Size¶
- setMinSize(number stage, number * size)¶
Change the min size from a particle system.
- Parameters
number – stage to be modified.
number – min size of the particle.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMinSize(stage,1)
else
print('Failed to load particle:','particle.ptl')
end
- getMinSize(number stage)¶
Get the min size from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
-min
size of the particle.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local minSize = tParticle:getMinSize(stage)
print('Current min size:',minSize)
else
print('Failed to load particle:','particle.ptl')
end
- setMaxSize(number stage, number size)¶
Change the max size from a particle system.
- Parameters
number – stage to be modified.
number – max size of the particle.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setMaxSize(stage,15.5)
else
print('Failed to load particle:','particle.ptl')
end
- getMaxSize(number stage)¶
Get the max life size from a particle system.
- Parameters
number – stage to retrieve.
- Returns
number
, max size of the particle.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local maxSize = tParticle:getMaxSize(stage)
print('Current max size:',maxSize)
else
print('Failed to load particle:','particle.ptl')
end
10.1.10. particle Stage¶
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local stage = tParticle:getStage()
print('Current stage:',stage)
else
print('Failed to load particle:','particle.ptl')
end
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local iTotalStage = tParticle:getTotalStage()
print('Total stage:',iTotalStage)
else
print('Failed to load particle:','particle.ptl')
end
- setStage(number stage)¶
Set the current stage to a particle system.
- Parameters
number – stage to be set.
Example:
local stage = 1
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setStage(stage)
else
print('Failed to load particle:','particle.ptl')
end
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local stage = tParticle:addStage()
print('Stage added at:',stage)
else
print('Failed to load particle:','particle.ptl')
end
- setStageTime(number stage, number time)¶
Set time for the stage in a particle system.
- Parameters
number – stage to be set.
number – time in seconds to change the stage.
Example:
local stage = 1
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
tParticle:setStageTime(stage,2) --2 seconds to change to next stage
else
print('Failed to load particle:','particle.ptl')
end
- getStageTime(number stage, number time)¶
Set time for the stage in a particle system.
- Parameters
number – stage to be set.
- Returns
number
-time
from stage.
Example:
local stage = 1
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
local iTime = tParticle:getStageTime(stage)
print('Time in the stage: ' .. iTime)
else
print('Failed to load particle:','particle.ptl')
end
10.1.11. particle Arise¶
Arise time is the time to show up since the particle system starts.For example, if is added 50 particle and the time is one second, then all 50 particle should be appear in the max one second.
- setAriseTime(number stage, number time)¶
Set the current arise time to a particle system.
- Parameters
number – stage to be set.
number – arise time to be set.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
tParticle:setAriseTime(stage,0.88)
else
print('Failed to load particle:','particle.ptl')
end
- getAriseTime(number stage)¶
Get the current arise time from particle system.
- Parameters
number – stage to be get.
- Returns
number
-arise time
from particle.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local ariseTime = tParticle:getAriseTime(stage)
print('Current arise time:',ariseTime)
else
print('Failed to load particle:','particle.ptl')
end
10.1.12. particle Invert color¶
- setInvertedColor(number stage, boolean r, boolean g, boolean b, boolean a)¶
Set individual inverted color to the current stage on a particle system.
- Parameters
number – stage to be set.
number – red color to be set.
number – green color to be set.
number – blue color to be set.
number – alpha color to be set.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
tParticle:setInvertedColor(stage,true,false,true,false)
else
print('Failed to load particle:','particle.ptl')
end
- getInvertedColor(number stage)¶
Get the current arise time from particle system.
- Parameters
number – stage to be get.
- Returns
boolean
,boolean
,boolean
,boolean
-r, g, b, a
indicating if is inverted.
Example:
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local ir,ig,ib,ia = tParticle:getInvertedColor(stage)
print(string .format('Inverted color R:%s, G:%s, B:%s, A:%s:',ir,ig,ib,ia))
else
print('Failed to load particle:','particle.ptl')
end
10.1.13. particle Total¶
- getTotalAlive¶
Retrieve the total of particles alive.
- Returns
number
-total
particle alive from system.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local total = tParticle:getTotalAlive()
print('Total particles alive in the system:',total)
else
print('Failed to load particle:','particle.ptl')
end
- getTotalParticle¶
Get the total particle capacity from particle system.
- Returns
number
-total
particle (dead + alive from system).
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local total = tParticle:getTotalParticle()
print('Total particles in the system:',total)
else
print('Failed to load particle:','particle.ptl')
end
- getTotalParticle(number stage)¶
Get the total particle available per stage from particle system.
- Parameters
number – stage to be get.
- Returns
number
-total
particle available to the stage indicated.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local stage = 1
local total = tParticle:getTotalParticle(stage)
print('Total particles in from stage selected:',total)
else
print('Failed to load particle:','particle.ptl')
end
- setTotalParticle(number stage, number total)¶
Set the total particle available per stage to particle system.
- Parameters
number – stage to be set.
number – total particle to the selected stage.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local stage = 1
tParticle:setTotalParticle(stage,100)
else
print('Failed to load particle:','particle.ptl')
end
10.1.14. particle getTexture¶
- getTexture¶
Get the current texture from particle system.
- Returns
string
-file name
from texture.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
print('Successfully loaded particle:','particle.ptl')
local texture_file_name = tParticle:getTexture()
print('Current texture used at particle:',texture_file_name)
else
print('Failed to loaded particle:','particle.ptl')
end
10.2. particle attributes¶
- alpha [read / write]
Enable or disable the alpha flag for a particle from current stage.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
tParticle.alpha = false
print('alpha:',tParticle.alpha)
else
print('Failed to load particle:','particle.ptl')
end
- revive [read / write]
Enable or disable the revive flag for a particle from current stage.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
tParticle.revive = false
print('revive:',tParticle.revive)
else
print('Failed to load particle:','particle.ptl')
end
- grow [read / write]
Enable or disable the grow flag for a particle from current stage.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
tParticle.grow = false
print('grow:',tParticle.grow)
else
print('Failed to load particle:','particle.ptl')
end
- segmented [read / write]
Enable or disable the segmented flag for a particle from current stage.
Example:
tParticle = particle:new('2dw')
if tParticle:load('particle.ptl',70,'*') then
tParticle.segmented = false
print('segmented:',tParticle.segmented)
else
print('Failed to load particle:','particle.ptl')
end
10.3. Creating particle programmatically¶
Follow some examples how to create particles programmatically.
For the first example, we will use a solid texture in red color.
mbm.setColor(0,0,0) --set background color to black
tParticle = particle:new('2dw')
tParticle:load('#FFFF0000') --red solid texture (alpha 1 red 1, green 0 blue)
tParticle:add(50)
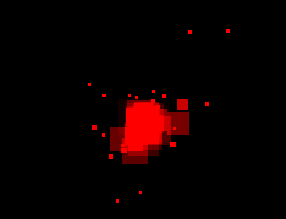
Figure 10.1 Simple particle with solid color red as texture.¶
Now, we will use the follow texture:
Particle using texture:
mbm.setColor(0,0,0) --set background color to black
tParticle = particle:new('2dw')
tParticle:load('particle_flame.png')
tParticle:add(50)
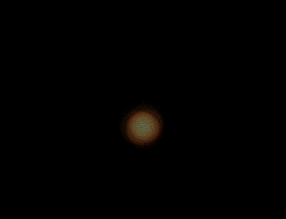
Figure 10.2 Simple particle using texture.¶
Particle using texture and changing the direction and speed:
mbm.setColor(0,0,0) --set background color to black
local stage = 1 --we only have one stage for this particle
tParticle = particle:new('2dw')
tParticle:load('particle_flame.png')
tParticle:setMinDirection(stage,-0.2,1,0)
tParticle:setMaxDirection(stage,0.2,1,0)
tParticle:setMinSpeed(stage,10)
tParticle:setMaxSpeed(stage,1000)
tParticle:add(1000)
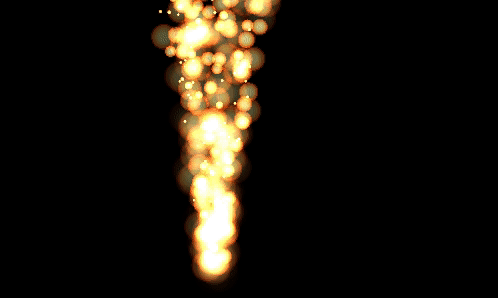
Figure 10.3 Changing the direction, speed and amount of particle.¶
Particle with 2 stages:
mbm.setColor(0,0,0) --set background color to black
local stage_1 = 1
--Set up for the first stage
tParticle:add(500) --500 particle for the first stage
tParticle:setMinSpeed(stage_1,10)
tParticle:setMaxSpeed(stage_1,1000)
tParticle:setMinLifeTime(stage_1,1)--at least one second for the life of particle
tParticle:setStageTime(stage_1,1)--one second of duration for the first stage
--Set up for the second stage
local stage_2 = tParticle:addStage()
tParticle:setMinDirection(stage_2,-0.2,1,0)
tParticle:setMaxDirection(stage_2,0.2,1,0)
tParticle:setMinSpeed(stage_2,10)
tParticle:setMaxSpeed(stage_2,1000)
tParticle:add(1000)--500 particle for the second stage
tParticle:restartAnim()--restart the animation since we have modified it
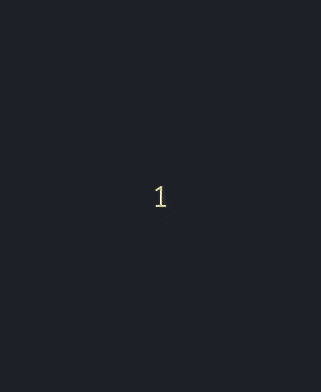
Figure 10.4 Particle with two stages¶
10.3.1. particle operator¶
Particle with different operator in the shader:
mbm.setColor(0,0,0) --set background color to black
local stage = 1 --we only have one stage for this particle
local total_particle = 300
local operator = { '+', '-', '/', '*'}
local x = {-300, -150, 0, 150}
tParticleArray = {} -- just to store 4 particle table
for i =1, #operator do
local tParticle = particle:new('2dw',x[i])
print('operator:',operator[i] )
tParticle:load('particle_flame.png',0,operator[i])
tParticle:setMinDirection(stage,-0.2,1,0)
tParticle:setMaxDirection(stage,0.2,1,0)
tParticle:setMinSpeed(stage,10)
tParticle:setMaxSpeed(stage,1000)
tParticle:add(total_particle)
table.insert(tParticleArray,tParticle)
end
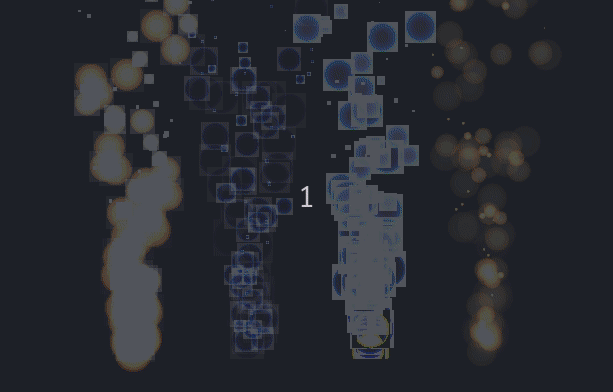
Figure 10.5 Four operator, +
, -
, /
, *
¶
10.3.2. particle fragment shader¶
The fragment shader (pixel shader) for particle is created in the moment of load.See how the options affect the shader:
precision mediump float;
uniform vec4 color;
uniform float enableAlphaFromColor;
varying vec2 vTexCoord;
uniform sampler2D sample0;
void main()
{
vec4 texColor;
vec4 outColor;
texColor = texture2D( sample0, vTexCoord );
if(enableAlphaFromColor > 0.5)
outColor.a = color.a;
else
outColor.a = texColor.a;
//The operator is used in the next line. the default if '*'
outColor.rgb = color.rgb * texColor.rgb;
//
//this line is the aditional code if supplied.
//
gl_FragColor = outColor;
}
10.3.3. particle vertex shader¶
The vertex shader used for the particle is:
attribute vec4 aPosition;
attribute vec2 aTextCoord;
uniform mat4 mvpMatrix;
varying vec2 vTexCoord;
void main()
{
gl_Position = mvpMatrix * aPosition;
vTexCoord = aTextCoord;
}
10.4. Saving particle to a binary file¶
It is possible to save any mesh with help of meshDebug. For that it is necessary to prepare the meshDebug based on our renderizable.
First, we have to create a temporary particle and set the values that we expect to have.
Then we create a meshDebug, add at least one frame and set it to
particle
type.Next, we copy the animations and shaders effect using the function copyAnimationsFromMesh.
Finally we just need specify the file name to save through the function save,.
Follow the example how to save a particle to a binary file:
1 mbm.setColor(0,0,0) --set background color to black 2 3 function createParticle() 4 5 local tParticle = particle:new('2dw') 6 tParticle:load('particle_flame.png') 7 8 local stage_1 = 1 9 10 --Set up for the first stage 11 tParticle:add(500) --500 particle for the first stage 12 13 tParticle:setMinSpeed(stage_1,10,10,0) 14 tParticle:setMaxSpeed(stage_1,1000,1000,0) 15 16 tParticle:setMinLifeTime(stage_1,1)--minimun alive for one second 17 tParticle:setMaxLifeTime(stage_1,2)--maximun alive for two seconds 18 19 tParticle:setStageTime(stage_1,1)--One second of duration 20 21 --Set up for the second stage 22 local stage_2 = tParticle:addStage() 23 24 tParticle:setMinDirection(stage_2,-0.2,1,0) 25 tParticle:setMaxDirection(stage_2,0.2,1,0) 26 27 tParticle:setMinSpeed(stage_2,10,10,0) 28 tParticle:setMaxSpeed(stage_2,1000,1000,0) 29 30 tParticle:add(1000)--500 particle for the second stage 31 32 tParticle:restartAnim()--restart the animation since we have modified it 33 return tParticle 34 end 35 36 function saveParticleToFile(fileName,tParticle) 37 38 --meshDebug is used to create dynamically the mesh in the engine. 39 --For particle it has to have at least one frame to be able to generate the mesh 40 local stride = 2 --stride only can be 3 or 2. it means (x,y,z) or (x,y) 41 local tMesh = meshDebug:new() --new mesh debug to store the information about our particle 42 local nFrame = tMesh:addFrame(stride) -- Add one frame with stride 2 (x,y) 43 local indexFrame = nFrame --(meshDebug uses 1 based index) 44 local indexSubset = 1 --first subset 45 local totalVertex = 3 --minimun 3 to build one triangle (otherwise the internal check of meshDebug will fail) 46 47 --To add vertex, first we need to add a subset 48 local nSubset = tMesh:addSubSet(indexFrame) --add one subset for the first frame 49 50 --we have to add one frame to set the texture and be able to save the mesh 51 --this frame has to have at least 3 vertex (one triangle) 52 if not tMesh:addVertex(indexFrame,indexSubset,totalVertex) then 53 print("Error on add vertex") 54 return false 55 end 56 57 --apply the texture from particle 58 if not tMesh:setTexture(indexFrame,indexSubset,tParticle:getTexture()) then 59 print("Error on set texture") 60 return false 61 end 62 63 tMesh:setType('particle') -- set it to particle type 64 65 if tMesh:copyAnimationsFromMesh(tParticle) then --copy animations and shaders, include stages. 66 local calcNormal,calcUv = false,false --dont wanna normal (neither recalc it) and recalc UV at all 67 if tMesh:save(fileName,calcNormal,calcUv) then 68 print("Particle created successfully ") 69 return true 70 else 71 print("Failed to create particle!") 72 return false 73 end 74 else 75 print("Failed to copy animations - stages, shaders...") 76 return false 77 end 78 end 79 80 81 local tParticle = createParticle() 82 83 if saveParticleToFile('particle.ptl',tParticle) then 84 tParticleFromFile = particle:new('2dw') 85 tParticleFromFile:load('particle.ptl') --all behavior already in place 86 end 87 88 tParticle:destroy()