Table of Contents
2. Scene¶
Most games use scene mechanism. This engine is no different.
When we have a new scene, the previous scene is destroyed and the new scene will become the current scene. It means that only the current scene exists when see the game.
This is how scenes in general works.
All we need, is to overload functions in the scene script that will be called by the engine.
There are a default scene internally. If any function does not exist, the default function is called.
Now, let’s see the main flow of a scene:
2.1. Main Scene’s flow¶
All functions described here can be overloaded.
2.1.1. onInitScene¶
- onInitScene¶
The engine will call this method only once during the execution’s script.
2.1.2. loop¶
- loop(delta)¶
Called each step. Delta indicate the interval passed since the last step.
- Parameters
number – delta time passed since the last step.
2.1.3. onEndScene¶
- onEndScene¶
The engine will call this method only once during the scene’ ending. The end of scene occurs when other scene is called or the application is shut down.
2.1.4. onRestore¶
- onRestore¶
Called on restore scene.
This usually happen when the device is lost.
2.2. Scene’s functions¶
2.2.1. onTouchDown¶
- onTouchDown(number key, number x, number y)¶
Called on touch down or click down on screen 2d.
- Parameters
number – key mouse click or finger on touch screen (start from 1).
number – x coordinate (left is 0).
number – y coordinate (up is 0).
2.2.2. onTouchUp¶
- onTouchUp(number key, number x, number y)¶
Called on touch up or click up on screen 2d.
- Parameters
number – key mouse click or finger on touch screen (start from 1).
number – x coordinate (left is 0).
number – y coordinate (up is 0).
2.2.3. onTouchMove¶
- onTouchMove(number key, number x, number y)¶
Called on touch move (mouse or finger) on screen 2d.
- Parameters
number – key mouse or finger on touch screen (start from 1).
number – x coordinate (left is 0).
number – y coordinate (up is 0).
2.2.4. onTouchZoom¶
- onTouchZoom(number zoom)¶
Called on zoom gesture or mouse scroll (values will be -1 and 1).
- Parameters
number – zoom values between -1 and 1.
2.2.5. onKeyDown¶
- onKeyDown(number key)¶
Called on key down.
- Parameters
number – key depends on operation system. the engine offers a function getCode and getKey translator for comparison.
2.2.6. onKeyUp¶
- onKeyUp(number key)¶
Called on key up.
- Parameters
number – key depends on operation system. the engine offers a function getCode and getKey translator for comparison.
2.2.7. onInfoJoystick¶
- onInfoJoystick(number player, number maxButtons, string deviceName, string extra)¶
Called on connect joystick. depends on operation system and joystick brand.
- Parameters
number – player indicate the player number.
number – maxButtons information about how many buttons are available in this joystick.
string – deviceName Joystick’s name. information from device.
string – extra It might have extra information about the joystick.
2.2.8. onKeyDownJoystick¶
- onKeyDownJoystick(number player, number key)¶
Called on joystick key down.
- Parameters
number – player indicate the player number.
number – key pressed on joystick.
2.2.9. onKeyUpJoystick¶
- onKeyUpJoystick(number player, number key)¶
Called on joystick key up.
- Parameters
number – player indicate the player number.
number – key pressed on joystick.
2.2.10. onMoveJoystick¶
- onMoveJoystick(number player, number lx, number ly, number rx, number ry)¶
Called on joystick move
- Parameters
number – player indicate the player number.
number – lx left pad on x axis.
number – ly left pad on y axis.
number – rx right pad on x axis.
number – ry right pad on y axis.
Attention
Although the Joystick’s functions had been tested including some joystick available on the market, there are a lot of them. Might not work properly on some devices.
2.2.11. onResizeWindow¶
- onResizeWindow¶
The engine will call this method whenever the window size has changed. It is up to the developer to rescale all scenes accordingly.
Note
When ‘onResizeWindow’ function is not available, the engine will restart the scene automatically since the scale has changed.
2.3. Example of Scene¶
Next we will use the following image to play with some scene’s example:
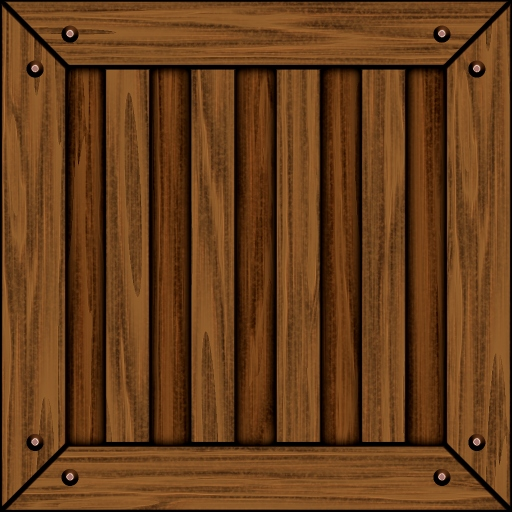
Figure 2.1 crate.png¶
--example of scene
function onInitScene()
tTexture = texture:new('2ds') --tTexture is global for the scene
tTexture:load('crate.png') --just load the texture (does not care if not found the texture)
tTexture:setScale(0.5,0.5) --adjust the scale
end
function onTouchMove(key,x,y)
tTexture:setPos(x,y) --move according to mouse or touch (we are ignoring the key)
end
function onTouchDown(key,x,y)
local tTextureTmp = texture:new('2ds',x,y)-- create a new local texture. ***this will be collected by GC***
tTextureTmp:load('crate.png') --Load it
tTextureTmp:setScale(0.5,0.5)--adjust the scale
tTextureTmp.az = tTexture.az -- adjust the rotation on z angle
tTexture.z = tTextureTmp.z -1 --bring to front
end
function loop(delta)
tTexture:rotate('z',math.rad(30)) -- rotate 30 degree per second on z angle
end
2.4. Example of Scene class style¶
--example of scene class style local tMyClass = {} tMyClass.onInitScene = function (self) self.tMyTexture = texture:new('2ds') --texture is part of tMyClass now self.tMyTexture:load('crate.png') -- just load the texture (does not care if not found the texture) self.tMyTexture:setScale(0.5,0.5) --adjust the scale end tMyClass.onTouchMove = function(self,key, x,y) self.tMyTexture:setPos(x,y) --move according to mouse or touch (we are ignoring the key) end tMyClass.loop= function(self,delta) self.tMyTexture:rotate('z',math.rad(30)) -- rotate 30 degree per second on z angle end tMyClass.onTouchDown = function(self,key, x,y) local tTextureTmp = texture:new('2ds',x,y)-- create a new local texture. ***this will be collected by GC*** tTextureTmp:load('crate.png') --Load it tTextureTmp:setScale(0.5,0.5)--adjust the scale tTextureTmp.az = self.tMyTexture.az -- adjust the rotation on z angle self.tMyTexture.z = tTextureTmp.z -1 --bring to front end local w, h = mbm.getSizeScreen() --[[ important return the class otherwise won't work ]] return tMyClass
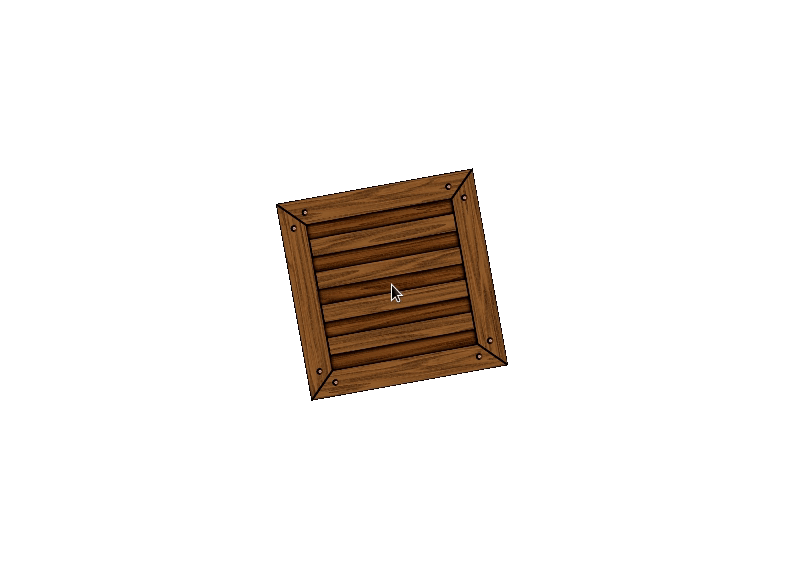
Figure 2.2 Both scene normal style and scene class style running.¶
Important
2.5. Example of Scene using coroutine¶
coroutine.yield
function from lua, there is an interruption and the engine does a loop of render.--example of scene using coroutine class style
local tMyClass = {}
tMyClass.onInitScene = function(self)
self.tTextures = {}
local iTotal = 91 --we put 91 just to understand how onProgress works (91 represent the end of the loop (100%))
for i=1,iTotal do
local x = i * 10
local y = i * 10
local tTexture = texture:new('2ds',x,y) --tTexture is local
tTexture:load('crate.png') --just load the texture (does not care if not found the texture)
tTexture:setScale(0.5,0.5) --adjust the scale
table.insert(self.tTextures,tTexture) --save tTexture to a table (will not be destroyed anymore)
--call coroutine.yield informing step and total of step (iStep , iTotal). our case (1,91), (2,91), (3,91), ...
--each loop will call onProgress where it could do some animation there.
--could be coroutine.yield() but in this case, will not call onProgress method
coroutine.yield(i,iTotal)
end
end
--This function only exist for class style and using coroutine
--This function is called each step if passed the progress to coroutine.yield (current and total steps)
tMyClass.onProgress = function(self,percent)
print(string.format('loaded: %.0f%%',percent))
end
tMyClass.onTouchMove = function(self,key,x,y)
self.tTextures[#self.tTextures]:setPos(x,y) --move according to mouse or touch (we are ignoring the key)
end
tMyClass.onTouchDown = function(self,key,x,y)
local tTexture = texture:new('2ds',x,y)-- create a new local texture.
tTexture:load('crate.png') --Load it
tTexture:setScale(0.5,0.5)--adjust the scale
tTexture.az = self.tTextures[#self.tTextures].az -- adjust the rotation on z angle
self.tTextures[#self.tTextures].z = tTexture.z -1 --bring to front
table.insert(self.tTextures,tTexture)
end
tMyClass.loop = function(self,delta)
for i=1,#self.tTextures do
local tTexture = self.tTextures[i]
tTexture:rotate('z',math.rad(30)) -- rotate 30 degree per second on z angle
end
end
--[[
important return the class otherwise won't work
]]
return tMyClass
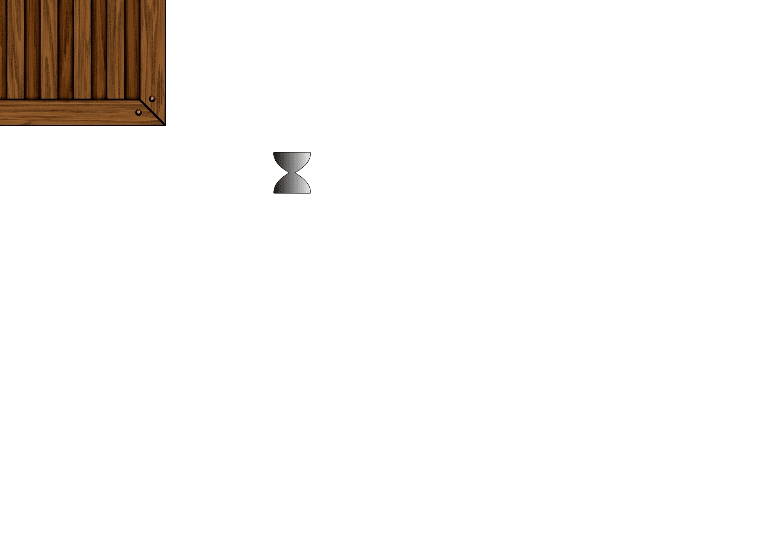
Figure 2.3 A scene class style running using coroutine.¶
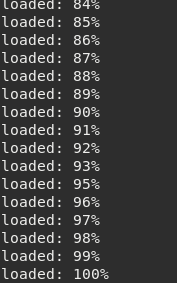
Figure 2.4 The output in the console.¶