Table of Contents
14. Table shape¶
A table shape inherit from renderizable and implement all methods as usual. Also each animation has a shader table by default which can be accessed by getShader method.
The main functionality of shape is to easily create a shape of circle, triangle or rectangle. Also is possible to create a free shape using the methods create and createIndexed.
The table shape is also capable to render in real time using the method onRender. To do that the frame must be created as dynamic mode option.
Note
The shape is not capable to store runtime information.
On lost device, the shape will lost its information, so, it must be reloaded on method onRestore.
Also is lost its texture and callback for onEndAnim and onEndFx.
14.1. shape methods¶
14.1.1. shape new¶
- new(string * world, number * x, number * y, number * z)¶
Create a new instance of a shape passing the world desired (detail) and position.
- Parameters
string – world can be
2ds
,2dw
or3d
.number – x position (optional).
number – y position (optional).
number – z position (optional).
- Returns
shape table.
Example:
tShape = shape:new('2dw')
14.1.2. shape create¶
- create(string type, number width, number * height, number * triangles, boolean * dynamic_buffer, string * nick_name)¶
Create a shape in runtime choosing among the pre defined option available.
The onRender callback can be used if dynamic_buffer is
true
.- Parameters
string – type pre defined.
circle
,rectangle
ortriangle
.number – width of shape.
number – height of shape.
number – triangles - default values
circle: 18
-rectangle: 2
,triangle: 1
.boolean – dynamic buffer option to render in runtime the shape using onRender method (optional).
string – nick name of shape (optional).
- Returns
string
- nick_name
Example:
tShapeCircle = shape:new('2dw', -100)
tShapeRectangle = shape:new('2dw', 0 )
tShapeTriangle = shape:new('2dw', 100)
local nickNameCircle = tShapeCircle:create('circle',100,100)
local nickNameRectangle = tShapeRectangle:create('rectangle',100,100)
local nickNameTriangle = tShapeTriangle:create('triangle',100,100)
if nickNameCircle then
print('Circle ' .. nickNameCircle .. ' successfully created!')
else
print('Failed to create a circle')
end
if nickNameRectangle then
print('Rectangle '.. nickNameRectangle .. ' successfully created!')
else
print('Failed to create a rectangle')
end
if nickNameTriangle then
print('Triangle '.. nickNameTriangle .. ' successfully created!')
else
print('Failed to create a triangle')
end
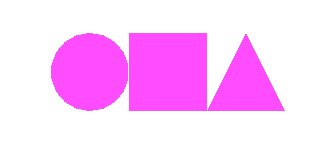
Figure 14.1 Three shapes created as result.¶
As is known, a shape is based on triangles and, to build a circle is not different. If is necessary to have a huge detail on circle, is recommended to create it using shader.
Example creating circle with different number of triangles
tShapeCircle_4 = shape:new('2dw', -200)
tShapeCircle_8 = shape:new('2dw', 0)
tShapeCircle_18 = shape:new('2dw', 200)
local nickNameCircle_4 = tShapeCircle_4:create ('circle',200,200, 4)
local nickNameCircle_8 = tShapeCircle_8:create ('circle',200,200, 8)
local nickNameCircle_18 = tShapeCircle_18:create('circle',200,200, 18)
if nickNameCircle_4 then
print('Circle ' .. nickNameCircle_4 .. ' with 4 triangle successfully created!')
else
print('Failed to create a circle')
end
if nickNameCircle_8 then
print('Circle ' .. nickNameCircle_8 .. ' with 8 triangle successfully created!')
else
print('Failed to create a circle')
end
if nickNameCircle_18 then
print('Circle ' .. nickNameCircle_18 .. ' with 18 triangle successfully created!')
else
print('Failed to create a circle')
end

Figure 14.2 Three circle created as result with different number of triangles. Although the first seems to be a square, it is a circle but with a minimum of triangles needed.¶
- create(table vertex, table* uv, table* normal, string * nick_name, string * mode_draw, string * mode_cull_face, string * mode_front_face_direction)¶
Create a shape in runtime supplying native coordinates.
- Parameters
table – vertex coordinates
x,y
orx,y,z
.table – uv coordinates
u,v
(optional).table – normal coordinates
x,y,z
(optional).string – nick name of shape (optional).
string – mode draw of shape (optional). see mode draw.
string – mode cull face of shape (optional). see cull face.
string – mode front face direction of shape (optional). see front face direction.
- Returns
string
- nick_name
Example:
-- Example: 2d
tShape = shape:new('2dw')
--letś draw a square of 400x400
-- First Triangle Second Triangle
local tVertex = {-200,-200, -200,200, 200,-200, 200,-200, -200,200, 200,200} -- (x,y) coordinates
local tUv = {0,1, 0,0, 1,1, 1,1, 0,0, 1,0}
local tNormal = nil --let the normal be calculated automatically
if tShape:create(tVertex,tUv,tNormal,'my-shape-nick-name') then
print('Shape successfully created!')
else
print('Failed to create a shape')
end
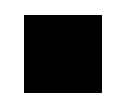
Figure 14.3 An square shape without texture as result¶
-- Example: 3d
tShape = shape:new('3d',0,0,500)
--letś draw a square of 400x400x0
-- First Triangle Second Triangle
local tVertex = {-200,-200,0, -200,200,0, 200,-200,0, 200,-200,0, -200,200,0, 200,200,0} -- (x,y) coordinates
local tUv = {0,1, 0,0, 1,1, 1,1, 0,0, 1,0}
local tNormal = nil --let the normal be calculated automatically
if tShape:create(tVertex,tUv,tNormal,'my-shape-nick-name','TRIANGLES', 'BACK','CW') then
print('Shape successfully created!')
else
print('Failed to create a shape')
end
14.1.3. shape createIndexed¶
- createIndexed(table vertex, table index, table* uv, table* normal, string * nick_name, string * mode_draw, string * mode_cull_face, string * mode_front_face_direction)¶
Create a shape in runtime supplying indexed native coordinates.
- Parameters
table – vertex coordinates
x,y
orx,y,z
.table – index array referent to vertex (one based).
table – uv coordinates
u,v
(optional).table – normal coordinates
x,y,z
(optional).string – nick name of shape (optional).
string – mode draw of shape (optional). see mode draw.
string – mode cull face of shape (optional). see cull face.
string – mode front face direction of shape (optional). see front face direction.
- Returns
string
- nick_name
Example: 2d
tShape = shape:new('2dw')
--letś draw a square of 400x400
-- 1 2 3 4
local tVertex = {-200,-200, -200,200, 200,-200, 200,200} -- (x,y) coordinates
local tUv = {0,1, 0,0, 1,1, 1,0 }
local tIndex = {1,2,3, 3,2,4 } -- one based
local tNormal = nil --let the normal be calculated automatically
if tShape:createIndexed(tVertex,tIndex,tUv,tNormal,'my-shape-nick-name','TRIANGLES', 'BACK','CW') then
print('Shape successfully created!')
else
print('Failed to create a shape')
end
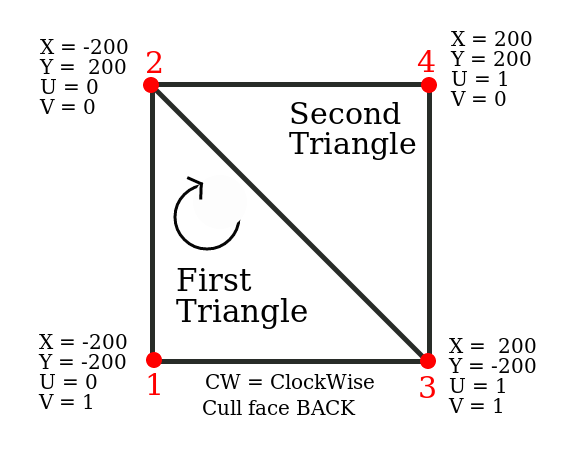
Figure 14.4 Rendering using triangle list, Clockwise and CullFace mode back (default of the engine).¶
14.1.4. shape createDynamicIndexed¶
- createDynamicIndexed(table vertex, table index, table* uv, table* normal, string * nick_name, string * mode_draw, string * mode_cull_face, string * mode_front_face_direction)¶
Create a dynamic shape in runtime supplying indexed native coordinates.
The onRender callback can be used after creating with this method.
- Parameters
table – vertex coordinates
x,y
orx,y,z
.table – index array referent to vertex (one based).
table – uv coordinates
u,v
(optional).table – normal coordinates
x,y,z
(optional).string – nick name of shape (optional).
string – mode draw of shape (optional). see mode draw.
string – mode cull face of shape (optional). see cull face.
string – mode front face direction of shape (optional). see front face direction.
- Returns
string
- nick_name
Example: 2d
tShape = shape:new('2dw')
--letś draw a square of 400x400
-- 1 2 3 4
local tVertex = {-200,-200, -200,200, 200,-200, 200,200} -- (x,y) coordinates
local tUv = {0,1, 0,0, 1,1, 1,0 }
local tIndex = {1,2,3, 3,2,4 } -- one based
local tNormal = nil --let the normal be calculated automatically
if tShape:createDynamicIndexed(tVertex,tIndex,tUv,tNormal,'my-shape-nick-name','TRIANGLES', 'BACK','CW') then
print('Shape successfully created!')
else
print('Failed to create a shape')
end
14.1.5. setColor¶
- setColor(number red, number green, number blue, number * alpha)¶
Set a solid color as texture.
- Parameters
number – red color. Values between 0.0 and 1.0
number – green color. Values between 0.0 and 1.0
number – blue color. Values between 0.0 and 1.0
number – alpha color. Values between 0.0 and 1.0
- Returns
boolean
- successfully applied.Example:
tShape = shape:new('2dw')
tShape:create('RECTANGLE',100,100)
local r,g,b, a = 1.0, 0.0, 0.0, 1.0
local ret = tShape:setColor(r,g,b,a)
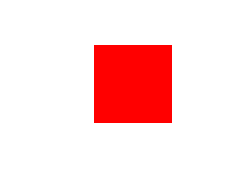
Figure 14.5 Setting red color for the shape¶
14.1.6. onRender¶
- onRender(tShape, vertex, normal, uv, index_read_only)¶
Shape is capable to render in run time if created with the option dynamic mode as mentioned at shape:create .
The data from callback are organized in
x, y, z
for vertex,nx, ny, nz
for normal andu, v
for texture coordinates.Also each table has a property
name
to identify when return from render callback.The first argument is the shape itself following from vertex table, normal table, uv table and index_buffer.
The index buffer is read only and one based. Do not need to return it.
Note
Passing
nil
to method onRender the callback is cancelled.- Parameters
table – shape instance of shape.
table – [x, y, x] vertex table.
table – [nx, ny, nz] normal table.
table – [u, v] texture coordinates.
table – [] array index buffer read only. (one based)
- Returns
table
vertex,table
normal,table
uv - ifnil
is returned the callback is cancelled. Not all arguments are necessary, just the one edited.Example how to access all members from callback:
mbm.setColor(0.7,0.7,0.7) --set background color to gray
local numberTriangle = 1
local isDynamic = true
tShape = shape:new('2dw')
nickName = tShape:create('Triangle',1000,1000,numberTriangle,isDynamic)
print('Shape Nick Name:' .. nickName)
function onRender(tShape,vertex,normal,uv,index_read_only)
print("--------------------")
print(" table (" .. vertex.name .. ')')
for i=1,#vertex do
print('x:' .. vertex[i].x .. ' y:' .. vertex[i].y .. ' z:' .. vertex[i].z)
end
print(" table (" .. normal.name .. ')')
for i=1,#normal do
print('nx:' .. normal[i].nx .. ' ny:' .. normal[i].ny .. ' nz:' .. normal[i].nz)
end
print(" table (" .. uv.name .. ')')
for i=1,#uv do
print('u:' .. uv[i].u .. ' v:' .. uv[i].v )
end
print("--------------------")
-- if does not return or return nil the callback is cancelled
return vertex,normal,uv
--is possible to choose what return because of the name in the table which identify it
-- example:
-- return uv, vertex
--
-- if return nil or does not return the callback is cancelled
-- example
-- return nil
end
--set the callback
tShape:onRender(onRender)
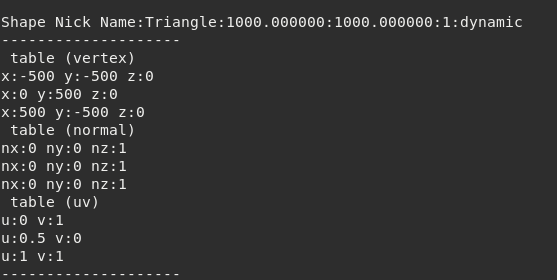
Figure 14.6 The output should be like this.¶
Warning
nil
or does not return, the callback is cancelled for onRender.